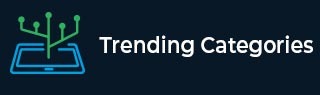
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What are printf conversion characters and their types?
The use of printf is to print out a string with no blank fields to be filled.
For example,
printf ("An ordinary string.."); printf ("Testing 1,2,3...");
The next simplest case that has been used before now is to print out a single integer number.
int number = 48; printf ("%d",number);
The two can be combined as shown below −
int number = 48; printf ("Some number = %d",number);
The result of this last example is to print out the following on the screen −
Some number = 48
Here is a list of the different letters for printf −
- d − signed denary integer
- u − unsigned denary integer
- x − hexadecimal integer
- o − octal integer
- s − string
- c − single character
- f − fixed decimal floating point
- e − scientific notation floating point
- g − use f or e, whichever is shorter
Example
Following is the C program for the use of printf conversion characters and types −
/* printf Conversion Characters and Types */ #include <stdio.h> main (){ int i = -10; unsigned int ui = 10; float x = 3.56; double y = 3.52; char ch = ’z’; char *string_ptr = "any old string"; printf ("signed integer %d
", i); printf ("unsigned integer %u
",ui); printf ("This is wrong! %u",i); printf ("See what happens when you get the "); printf ("character wrong!"); printf ("Hexadecimal %x %x
",i,ui); printf ("Octal %o %o
",i,ui); printf ("Float and double %f %f
",x,y); printf (" ditto %e %e
",x,y); printf (" ditto %g %g
",x,y); printf ("single character %c
",ch); printf ("whole string -> %s",string_ptr); }
Output
When the above program is executed, it produces the following result −
signed integer -10 unsigned integer 10 This is wrong! 4294967286See what happens when you get the character wrong!Hexadecimal fffffff6 a Octal 37777777766 12 Float and double 3.560000 3.520000 ditto 3.560000e+000 3.520000e+000 ditto 3.56 3.52 single character z whole string -> any old string
Advertisements