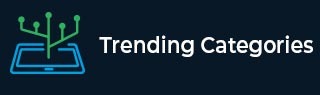
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What are constants in Kotlin and how to create them?
In every programming language, we need some variable whose value will never change thoroughout the program. In Kotlin too, we have a keyword to create such a variable whose value will remain as constant throughout the program. In order to declare a value as constant, we can use the "const" keyword at the beginning. In this article, we will learn how we can declare a variable as constant in a different way.
Example: Top level declaration
Example
Kotlin const variable can be declared at the top of the programming language and it can be used throughout the file scope.
private const val My_TOP_LEVEL_CONST_VAL = "Type 1--> Example of Top Level Constant Value" fun main() { println(My_TOP_LEVEL_CONST_VAL); }
Output
It will produce the following output −
Type 1--> Example of Top Level Constant Value
Example: Local constant
Like any other programming language, in Kotlin too, we can declare a local constant value and it will be blocked with in the specified scope. In the following example, we will create a local constant value.
Example
fun main() { val MY_LOCAL_CONST="Type 2-->Example of local const value" println(MY_LOCAL_CONST); }
Output
It will produce the following output −
Type 2-->Example of local const value
Example: Companion object const
Kotlin also provides an option to create a const function in the companion object. This is not recommended as per the recent programming architecture, because a companion object by default creates its own getter() and setter() methods which may cause performance issues.
Example
fun main() { println(Student.MY_CONSTANT); } class Student(){ companion object{ const val MY_CONSTANT = "Type 3--> Using companion Object" } }
Output
It will produce the following output −
Type 3--> Using companion Object
Example: Object declaration and direct call
A Constant variable can also be declared inside an object class. Later, this variable can be used by different means inside the program.
Example
fun main() { println(MyConstant.Const_USING_OBJECT_CLASS); } object MyConstant { const val Const_USING_OBJECT_CLASS = "Type 4-->Example of const using object class" }
Output
It will produce the following output −
Type 4-->Example of const using object class