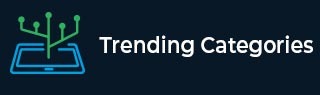
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What are Async Streams in C# 8.0?
C# 8.0 introduces async streams, which model a streaming source of data. Data streams often retrieve or generate elements asynchronously.
The code that generates the sequence can now use yield return to return elements in a method that was declared with the async modifier.
We can consume an async stream using an await foreach loop.
This below Syntax
static IEnumerable<string> Message(){ yield return "Hello!"; yield return "Hello!"; } Can be replaced by IAsyncEnumerable static async IAsyncEnumerable<string> MessageAsync(){ await Task.Delay(2000); yield return "Hello!"; await Task.Delay(2000); yield return "Hello!"; }
Example
class Program{ public static async Task Main(){ await foreach (var item in MessageAsync()){ System.Console.WriteLine(item); } Console.ReadLine(); } static async IAsyncEnumerable<string> MessageAsync(){ await Task.Delay(2000); yield return "Hello!"; await Task.Delay(2000); yield return "Hello!"; } }
Output
Hello! Hello!
Advertisements