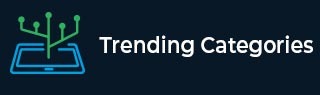
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
What are abstract classes in C#?
Abstract classes contain abstract methods, which are implemented by the derived class. The derived classes have more specialized functionality.
The following is an example showing the usage of abstract classes in C#.
Example
using System; namespace Demo { abstract class Shape { public abstract int area(); } class Rectangle: Shape { private int length; private int width; public Rectangle( int a = 0, int b = 0) { length = a; width = b; Console.WriteLine("Length of Rectangle: "+length); Console.WriteLine("Width of Rectangle: "+width); } public override int area () { return (width * length); } } class RectangleTester { static void Main(string[] args) { Rectangle r = new Rectangle(14, 8); double a = r.area(); Console.WriteLine("Area: {0}",a); Console.ReadKey(); } } }
Output
Length of Rectangle: 14 Width of Rectangle: 8 Area: 112
Our abstract class above is −
abstract class Shape { public abstract int area(); }
The following are the rules about abstract classes.
- You cannot create an instance of an abstract class
- You cannot declare an abstract method outside an abstract class
- When a class is declared sealed, it cannot be inherited, abstract classes cannot be declared sealed.
Advertisements