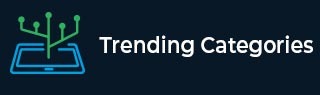
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Ways to paint stairs with two colors such that two adjacent are not yellow in C++
We are given n stairs and 2 colors (red and yellow) with which these stairs are to be painted. Our task is to count the number of ways in which we can paint the stairs such that no two consecutive steps will be yellow-colored.
Let’s take an example to understand the problem,
Input
3
Output
5
Explanation
The ways in which stairs can be painted are YRY, RYR, YRR, RRY, RRR. here R denotes red color, Y denotes yellow color.
To solve this problem, let’s see the number of ways the stairs can be painted.
N = 1, ways(1) = 2 : R, Y
N = 2, ways(2) = 3 : RY, YR, RR
N = 3, ways(3) = 5 : RYR, YRY, RRY, YRR, RRR
N = 4, ways(4) = 8 : YRYR, RYRY, RYRR, YRRY, YRRR, RRYR, RRRR, RRRY.
So from these cases, we can derivate that this is a Fibonacci Series starting with 2 as first element and 3 as second.
Program to illustrate the working of our logic,
Example
#include <iostream> using namespace std; int colorSteps(int n) { int first = 2; int next = 3; for (int i = 3; i <= n; i++) { next = first + next; first = next - first; } return next; } int main(){ int n = 6; cout<<"Number of ways to color "<<n<<" steps is "<<colorSteps(n); return 0; }
Output
Number of ways to color 6 steps is 21
Advertisements