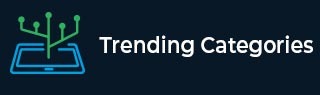
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Ways to paint N paintings such that adjacent paintings don’t have same colors in C programming
In this problem, you are given N painting and we have m color that we can make paintings with and we need to find the number of ways in which we can draw the painting such that none of the same color paintings are to each other.
The program’s output can have very large values and handing these values is a bit problem so we will calculate its answer in standard modulo 109 +7.
The formula to find the number ways is :
Ways = n*(m-1)(n-1)
Example to describe the problem, this will need the number of paintings n and number of colors m :
Input
n = 5 ,m = 6
Output
3750
Example
#include <iostream> #include<math.h> #define modd 1000000007 using namespace std; unsigned long find(unsigned long x, unsigned long y, unsigned long p) { unsigned long res = 1; x = x % p; while (y > 0) { if (y & 1) res = (res * x) % p; y = y >> 1; x = (x * x) % p; } return res; } int ways(int n, int m) { return find(m - 1, n - 1, modd) * m % modd; } int main() { int n = 5, m = 6; cout<<"There are"<<ways(n, m)<<"ways"; return 0; }
Output
There are 3750 ways
Advertisements