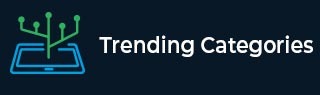
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Wait for user input to start a sketch in Arduino
A problem faced by several people using Arduino, or any microcontroller board for that matter, is that you may forget to start the Serial Monitor before programming the board, and miss some print statements by the time you open the Serial Monitor.
One way to overcome this is to start the sketch only after an input is received from the user, via the Serial Monitor. This will ensure that you don’t miss any prints on the Serial Monitor because of the delay in starting the Serial Monitor.
Example
void setup() { // put your setup code here, to run once: Serial.begin(9600); Serial.println(); Serial.println("Please send a character to start the sketch"); while (Serial.available() == 0) { Serial.print("."); delay(500); } while (Serial.available() > 0) { Serial.read(); //Empty the read buffer } } void loop() { // put your main code here, to run repeatedly: Serial.println("Looping!"); delay(1000); }
As you can see, the sketch first waits for the user input. As soon as the user input is received, the sketch clears the read buffer, and then proceeds with the rest of the tasks.
Output
The Serial Monitor Outputs show that
Please note that waiting for user input should only be done in the test code, and not in the production code, where there is no possibility of getting the user input.