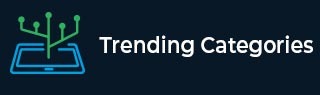
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Voca: The Ultimate Javascript library for String Manipulation
Voca is a JavaScript library that is used for manipulating strings. In this tutorial, we will take multiple examples to show how you can use the different functions available in Voca.
Features of Voca
Before we see all the examples, let's highlight some features that Voca brings to the table −
It provides a multitude of functions that can be used to manipulate, query, escape, format strings.
It also provides a detailed and searchable documentation.
It supports a wide range of environments like Node,js, Safari 7+, Chrome, Firefox etc.
It doesn't require any dependencies
How to Install Voca?
Now that we know the features of Voca.js, let's see how you can install it on our local machine. To install Voca, run the following command in the terminal −
npm install voca
Once you run the above command in the terminal, a "package.json" file along with a "package-lock.json" and a "node_modules" folder will be created. Now, we are ready to use Voca functions in our code.
Since we will be talking about a lot of functions of Voca, it's better if we split them into different common categories.
Changing the String Case
The first category of examples that we will explore is the case, in which we change the case of a particular text.
camelCase() Function
The camelCase() function is used when we want to convert a text into its camel case representation. Consider the code shown below.
const v = require('voca'); let companyName = 'tutorials point'; console.log("Input Text -", companyName); console.log('Camel Case -', v.camelCase(companyName));
To run the above code, first save it with the name "index.js" and then run the following command.
node index.js
It will produce the following output
Input Text - tutorials point Camel Case - tutorialsPoint
capitalize() Function
The capitalize() function is used when we want to convert a text into its capitalization representation. Consider the code shown below.
const v = require('voca'); let companyName = 'tutorials point'; console.log('Input Text –', companyName); console.log('Capitalize –', v.capitalize(companyName));
It will produce the following output
Input Text – tutorials point Capitalize – Tutorials point
decapitalize() Function
The decapitalize() function is used when we want to convert a text to its decapitalized representation. Consider the code shown below.
const v = require('voca'); let companyName = 'Tutorials point'; console.log('Input - ', companyName); console.log('Decapitalize -', v.decapitalize(companyName));
It will produce the following output
Input - Tutorials point Decapitalize - tutorials point
kebabCase() Function
The kebabCase() function is used when we want to convert a text to its kebabCase representation. Consider the code shown below.
const v = require('voca'); let companyName = 'tutorials point'; console.log('Input -', companyName); console.log('KebabCase -', v.kebabCase(companyName));
It will produce the following output
Input - tutorials point KebabCase - tutorials-point
snakeCase() Function
The snakeCase() function is used when we want to convert a text to its snakeCake representation. Consider the code shown below.
const v = require('voca'); let companyName = 'tutorials point'; console.log('Input -', companyName); console.log('snakeCase -', v.snakeCase(companyName));
It will produce the following output
Input - tutorials point snakeCase - tutorials_point
lowerCase() Function
The lowerCase() function is used when we want to convert a text to its lowerCase representation. Consider the code shown below.
const v = require('voca'); let companyName = 'TUTORIALS point'; console.log('Input -', companyName); console.log('LowerCase -', v.lowerCase(companyName));
It will produce the following output
Input - TUTORIALS point LowerCase - tutorials point
swapCase() Function
The swapCase() function is used when we want to convert a text to its swapCase representation. Consider the code shown below.
const v = require('voca'); let companyName = 'tutorials point'; console.log('Input -', companyName); console.log('SwapCase -', v.swapCase(companyName));
It will produce the following output
Input - tutorials point SwapCase - TUTORIALS POINT
titleCase() Function
The titleCase() function is used when we want to convert a text to its titleCase representation. Consider the code shown below.
const v = require('voca'); let companyName = 'tutorials point'; console.log('Input -', companyName); console.log('TitleCase -', v.titleCase(companyName));
It will produce the following output
Input - tutorials point TitleCase - Tutorials Point
upperCase() Function
The upperCase() function is used when we want to convert a text to its upperCase representation. Consider the code shown below.
const v = require('voca'); let companyName = 'tutorials point'; console.log('Input -', companyName); console.log('UpperCase -', v.upperCase(companyName));
It will produce the following output
Input - tutorials point UpperCase - TUTORIALS POINT
Chaining Using Voca
Chaining is when we are able to chain multiple functions one after the another. Consider the code shown below.
const v = require('voca'); let str = 'Tutorials Point is Awesome!'; console.log('Creating a chain object -', v(str).lowerCase().words()); console.log('Chaining and Wrapping -', v.chain(str).lowerCase().words().value());
It will produce the following output
Creating a chain object - [ 'tutorials', 'point', 'is', 'awesome' ] Chaining and Wrapping - [ 'tutorials', 'point', 'is', 'awesome' ]
Chopping Using Voca
Chopping includes string manipulation functions like charAt(), first(), last(), etc.
charAt() Function
The charAt() function is used when we want to get a character that is present at a particular index. Consider the code shown below.
const v = require('voca'); let thingsILove = 'Formula1-Football-Leetcode-Sleeping'; console.log('Input String -', thingsILove); console.log('charAt 10th index -', v.charAt(thingsILove, 10)); console.log('charAt 7th index -', v.charAt(thingsILove, 7));
It will produce the following output
Input String - Formula1-Football-Leetcode-Sleeping charAt 10th index - o charAt 7th index - 1
first() Function
The first() function is used when we want to extract the first characters from a text. Consider the code shown below.
const v = require('voca'); let thingsILove = 'Formula1-Football-Leetcode-Sleeping'; console.log('Input -', thingsILove); console.log('first -', v.first(thingsILove)); console.log('first -', v.first(thingsILove, 8));
It will produce the following output
Input - Formula1-Football-Leetcode-Sleeping first - F first - Formula1
last() Function
The last() function is used when we want to extract the last characters from a text. Consider the code shown below.
const v = require('voca'); let thingsILove = 'Formula1-Football-Leetcode-Sleeping'; console.log('Input -', thingsILove); console.log('last -', v.last(thingsILove)); console.log('last -', v.last(thingsILove, 8));
It will produce the following output
Input - Formula1-Football-Leetcode-Sleeping last - g last - Sleeping
Slicing Using Voca
The slice() function is used when we want to extract a slice from the text. Consider the code shown below.
const v = require('voca'); console.log(v.slice('Delhi', 1)); console.log(v.slice('India', -4));
It will produce the following output
elhi ndia
Extracting a Substring Using Voca
The substring() function is used when we want to extract a substring from the text. The last element will also be included. Consider the code shown below.
const v = require('voca'); console.log(v.substring('Delhi', 3)); console.log(v.substring('India', 2, 4));
It will produce the following output
hi di
Count Function in Voca
The count() function is used when we want to count the number of words present in a text. Consider the code shown below.
const v = require('voca'); console.log(v.count('Delhi'));
It will produce the following output
5
Counting the Number of Substrings
The countSubstrings() function is used when we want to count the number of substrings present in a text. Consider the code shown below.
const v = require('voca'); console.log(v.countSubstrings('India is beautiful. India is huge!', 'India'));
It will produce the following output
2
Index Functions in Voca
In the index related methods, we will use the indexOf() function that is mainly used when we want to find at what particular index a particular string is present in a text. Consider the example shown below.
console.log(v.indexOf('India', 'n')); console.log(v.indexOf('India', 'p')); console.log(v.indexOf('Leetcode', 'e'));
It will produce the following output
1 -1 1
Observe that in the second case, the letter "p" is not there in the input string which is searched, hence it returns "-1" as the output.
Insert Function in Voca
The insert() function is used when we want to insert a particular text in between a text. Consider the example shown below.
const v = require('voca'); console.log(v.insert('cde','o',1));
It will produce the following output
code
It inserted the letter "o" at the position "1" in the given string.
Repeat Function in Vocac
The repeat() function is used when we want to repeat a particular text a number of times. Consider the example shown below.
const v = require('voca'); console.log(v.repeat('a', 3));
It will produce the following output
aaa
Reversing a String Using Voca
The reverse() function is used when we want to reverse a particular text. Consider the example shown below.
const v = require('voca'); console.log(v.reverse('apple'));
It will produce the following output
elppa
Trimming a String Using Voca
The trim() function is used when we want to trim a particular text from both the left side and the right side of the text. Consider the example shown below.
const v = require('voca'); console.log(v.trim(' an apple falling too down under '));
In the above example, we can see that there is some extra space (whitespace) present on both the sides of the text and we can remove that with the help of the trim() function available in the Voca package.
It will produce the following output
an apple falling too down under
Checking if a String is Empty
The isEmpty() function is used when we want to check whether a particular text is empty or not. Consider the example shown below.
const v = require('voca'); console.log(v.isEmpty(''));
It will produce the following output
true
It returns "true" as the input string is empty.
Checking if a String is of Numeric Type
The isNumeric() function is used when we want to check whether a particular text is of numeric type or not. Consider the example shown below.
const v = require('voca'); console.log(v.isNumeric('Hey there')); console.log(v.isNumeric(3));
It will produce the following output
false true
Checking if a Text is of String Type
The isString() function is used when we want to check whether a particular text is of string type or not. Consider the example shown below.
const v = require('voca'); console.log(v.isString('Hey there')); console.log(v.isString(12345));
It will produce the following output
true false
It returns "true" in the first case as the input text is of String type. In the second case, the input text is an Integer type, hence it returns "false".
startsWith Function in Voca
The startsWith() function is used when we want to check whether a particular text begins with a text or not. Consider the example shown below.
const v = require('voca'); console.log(v.startsWith('Hey there, join us?', 'Hey'));
It will produce the following output
true
The input string starts with the substring "Hey", hence it returns "true".
endsWith Function in Voca
The endsWith() function is used when we want to check whether a particular text ends with a text or not. Consider the example shown below.
const v = require('voca'); console.log(v.endsWith('Hey there, join us?', 'us?'));
It will produce the following output
true
Here, we are checking whether the input string ends with the substring "us?". It returns "true" as the input string indeed ends with the given substring.
includes() Function in Voca
The includes()
function is used when we want to check whether a particular text contains a specified text inside it or not. Consider the example shown below.const v = require('voca'); console.log(v.includes('Hey there, join us?', 'oin'));
It will produce the following output
true
Here, the input string contains the given substring "oin", hence it returns "true".
Conclusion
In this tutorial, we used several examples to demonstrate how you can utilize some of the popular string manipulation functions of Voca.