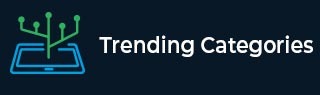
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Variable in subclass hides the variable in the super class in Java
Sometimes the variable in the subclass may hide the variable in the super class. In that case, the parent class variable can be referred to using the super keyword in Java.
A program that demonstrates this is given as follows:
Example
class A { char ch; } class B extends A { char ch; B(char val1, char val2) { super.ch = val1; ch = val2; } void display() { System.out.println("In Superclass, ch = " + super.ch); System.out.println("In subclass, ch = " + ch); } } public class Demo { public static void main(String args[]) { B obj = new B('B', 'E'); obj.display(); } }
Output
In Superclass, ch = B In subclass, ch = E
Now let us understand the above program.
The class A contains a data member ch. The class B uses the extends keyword to derive from class A. It also contains a data member ch and constructor B().
The ch variable in the subclass(B) hides the ch variable in the super class(A). So the parent class variable ch is referred to using the super keyword in constructor B(). The method display() in class B prints the values of ch in subclass and superclass. A code snippet which demonstrates this is as follows:
class A { char ch; } class B extends A { char ch; B(char val1, char val2) { super.ch = val1; ch = val2; } void display() { System.out.println("In Superclass, ch = " + super.ch); System.out.println("In subclass, ch = " + ch); } }
In the main() method in class Demo, an object obj of class B is created. Then the method display() is called. A code snippet which demonstrates this is as follows:
public class Demo { public static void main(String args[]) { B obj = new B('B', 'E'); obj.display(); } }