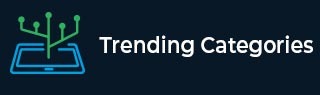
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Typing and Deleting Effect with JavaScript and CSS
With the help of CSS animations, we can create a typewriter typing and deleting effect using JavaScript. The infinite effect is also set. The custom function will get called and the words will get display with the effect. At the end, those words will get deleted using another custom function.
Set a div for the text and cursor
First, a parent div container is set with the <p> element. One of the <p> will have text and another the cursorL
<div> <p id="text"></p> <p>|</p> </div>
Style the <p> element
A professional font is set for the text −
p { font-family: "Courier Monospace"; font-size: 2em; color: red; }
The :last-of-type selector
To match every element that is the last child of its parent, use the :last-of-type selector. Set the animation for the last <p> element of its parent −
p:last-of-type { animation: type 0.33s infinite; }
Above, the animation name is type, which is set with an animation rule using keyframes. Here, the opacity is set using the opacity property −
@keyframes type{ to { opacity: .0; } }
Initial words
The following words are set and would be animated with the typing effect when the page loads −
const words = ["Nulla", "facilisi", "Cras sed odio sed leo laoreet molestie id non purus.."];
The page load
The custom function gets called when the page loads for typing −
function typeNow() { let word = words[i].split(""); var loopTyping = function() { if (word.length > 0) { document.getElementById('text').innerHTML += word.shift(); } else { deleteNow(); return false; }; counter = setTimeout(loopTyping, 200); }; loopTyping(); };
For the delete effect −
function deleteNow() { let word = words[i].split(""); var loopDeleting = function() { if (word.length > 0) { word.pop(); document.getElementById('text').innerHTML = word.join(""); } else { if (words.length > (i + 1)) { i++; } else { i = 0; }; typeNow(); return false; }; counter = setTimeout(loopDeleting, 200); }; loopDeleting(); };
Timeout
For the typing and deleting effect to work correctly, the setTimeout() function is called. The below works for both the events i.e., when a letter is typed and deleted −
counter = setTimeout(loopDeleting, 200);
Example
The following example shows this effect −
<!DOCTYPE html> <html> <head> <style> div { display: flex; margin: 4%; padding: 2%; box-shadow: inset 0 0 12px blue; } p { font-family: "Courier Monospace"; font-size: 2em; color: red; } p:last-of-type { animation: type 0.33s infinite; } @keyframes type{ to { opacity: .0; } } </style> </head> <body> <div> <p id="text"></p> <p>|</p> </div> <script> const words = ["Nulla", "facilisi", "Cras sed odio sed leo laoreet molestie id non purus.."]; let i = 0; let counter; function typeNow() { let word = words[i].split(""); var loopTyping = function() { if (word.length > 0) { document.getElementById('text').innerHTML += word.shift(); } else { deleteNow(); return false; }; counter = setTimeout(loopTyping, 200); }; loopTyping(); }; function deleteNow() { let word = words[i].split(""); var loopDeleting = function() { if (word.length > 0) { word.pop(); document.getElementById('text').innerHTML = word.join(""); } else { if (words.length > (i + 1)) { i++; } else { i = 0; }; typeNow(); return false; }; counter = setTimeout(loopDeleting, 200); }; loopDeleting(); }; typeNow(); </script> </body> </html>