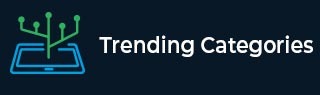
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Types of iterators in Ruby
In Ruby, we have multiple types of iterators available to us. We will learn about the most common ones in this article, one by one.
Each Iterator
Using this iterator, you can iterate over an array or a hash, returning each element as it is returned.
Example 1
Consider the code shown below
# each iterator example (0..10).each do |itr| puts itr end
Output
0 1 2 3 4 5 6 7 8 9 10
Times Iterator
This iterator implants a loop with a specific number of iterations. The loop starts from zero and runs until it gets one less than the specified number.
Example 2
# time iterator example 5.times do |itr| puts itr end
Output
0 1 2 3 4
Collect Iterator
The collect iterator returns the elements of the collection in an array or hash, regardless of the type of collection it is.
Example 3
# collect iterator example arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12] res = arr.collect{ |y| (4 * y) } puts res
Output
4 8 12 16 20 24 28 32 36 40 44 48
Advertisements