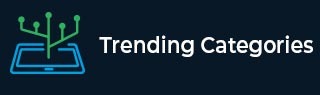
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Type.Equals() Method in C#
The Type.Equals() method in C# determines if the underlying system type of the current Type is the same as the underlying system type of the specified Object or Type.
Syntax
public virtual bool Equals (Type o); public override bool Equals (object o);
Above, the parameters are the object whose underlying system type is to be compared with the underlying system type of the current Type.
Let us now see an example to implement the Type.Equals() method −
using System; public class Demo { public static void Main(string[] args) { Type val1 = typeof(System.UInt16); Type val2 = typeof(System.Int32); Console.WriteLine("Are both the types equal? "+val1.Equals(val2)); } }
Output
This will produce the following output −
Are both the types equal? False
Let us now see another example to implement the Type.Equals() method −
Example
using System; using System.Reflection; public class Demo { public static void Main(string[] args) { Type type = typeof(String); Object obj = typeof(String).GetTypeInfo(); Type type2 = obj as Type; if (type2 != null) Console.WriteLine("Both types are equal? " +type.Equals(type2)); else Console.WriteLine("Cannot cast!"); } }
Output
This will produce the following output −
Both types are equal? True
Advertisements