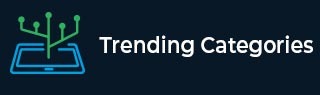
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
The remove() method of Java AbstractSequentialList class
The remove() method of the AbstractSequentialList class removes the element at the specified position in this list.
The syntax is as follows
E remove(int index)
Here, index is the index of the element to be removed. To work with the AbstractSequentialList class in Java, you need to import the following package
import java.util.AbstractSequentialList;
The following is an example to implement AbstractSequentialList remove() method in Java
Example
import java.util.LinkedList; import java.util.AbstractSequentialList; public class Demo { public static void main(String[] args) { AbstractSequentialList<Integer> absSequential = new LinkedList<>(); absSequential.add(25); absSequential.add(32); absSequential.add(40); absSequential.add(55); absSequential.add(60); absSequential.add(70); absSequential.add(90); System.out.println("Elements in the AbstractSequentialList = "+absSequential); absSequential.remove(3); absSequential.remove(5); System.out.println("Updated AbstractSequentialList = "+absSequential); } }
Output
Elements in the AbstractSequentialList = [25, 32, 40, 55, 60, 70, 90] Updated AbstractSequentialList = [25, 32, 40, 60, 70]
Advertisements