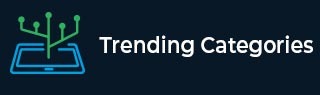
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
The lastIndex property in JavaScript
In this tutorial, we will learn about the lastIndex property in javascript. In addition to this, we will also learn about how we can use it to manage matches within a string
The lastIndex property is a property given by regex objects in JavaScript. It represents the index at which the next search will start within a string passed in the regex, or in simple terms, the index at which to start the next match in a string. When using a regex object with methods like exec(), compile(), etc, the lastIndex property is automatically updated to the index after the last match. It, then ultimately, allows us to perform the later searches within a string, continuing from where the last match ended.
Let’s understand the above concept with the help of some examples.
Example 1
To match the word "tutorials_point" throughout the string, we will create a regex pattern with the syntax /tutorials_point/g in this example. We will then use a while loop with the exec() method to find all matches. With each iteration, we log the index of the match and the value of lastIndex to the browser console.
Filename: index.html
<html lang="en"> <head> <title>The lastIndex property in JavaScript</title> </head> <body> <h3>The lastIndex property in JavaScript</h3> <pre id="code" class=”code”></pre> <script> const code = document.getElementById("code"); const regex = /tutorials_point/g; const str = "hello world hello tutorials_point"; let match; while ((match = regex.exec(str))) { console.log(`Match found at index ${match.index}`); console.log(`Next search will start at index ${regex.lastIndex}`); } code.innerHTML = `let regexToMatch = /tutorials_point/g; const str = "hello world hello tutorials_point"; let match; while ((match = regex.exec(str))) { console.log(\`Match found at index \${match.index}\`); console.log(\`Next search will start at index \${regex.lastIndex}\`); } <br> <br> // Output: <br> // Match found at index 18 <br> // Next search will start at index 33 <br>` code.innerHTML += `output: Match found at index 18 <br> Next search will start at index 33`; </script> </body> </html>
Output
Example 2
In this example, the lastIndex property of the regex object will be manually set to 0 before the loop is initiated. By doing this, one can be guaranteed that the search will start at the beginning of the string independent of any prior matches or values that lastIndex may have kept.
Filename: index.html
<html lang="en"> <head> <title>The lastIndex property in JavaScript</title> </head> <body> <h3>The lastIndex property in JavaScript</h3> <pre id="code"></pre> <script> const code = document.getElementById("code"); const regex = /tutorials_point/g; const str = "hello world hello tutorials_point"; regex.lastIndex = 0; // Resetting the lastIndex let match; while ((match = regex.exec(str))) { console.log(`Match found at index ${match.index}`); console.log(`Next search will start at index ${regex.lastIndex}`); } code.innerHTML = `const regex = /tutorials_point/g; <br> const str = 'hello world hello tutorials_point'; <br> regex.lastIndex = 0; // Resetting the lastIndex <br> let match; <br> while ((match = regex.exec(str))) { <br> console.log(\`Match found at index 18); <br> console.log(\`Next search will start at index 33); <br> } `; </script> </body> </html>
Output
Example 3
ITo match any string of digits, we will build a regex pattern (d+) for this example. To build a capturing group, or to match and capture one or more than one digit included in the string, the (d+) pattern is surrounded in parenthesis. We will use the “g” flag to perform a global search. This allows us to find all occurrences of numbers in the string.
Filename: index.html
<html lang="en"> <head> <title>The lastIndex property in JavaScript</title> </head> <body> <h3>The lastIndex property in JavaScript</h3> <pre id="code"></pre> <script> const code = document.getElementById("code"); const regex = /(\d+)/g; const str = "2022 is almost over, and 2023 is around the corner."; let match; while ((match = regex.exec(str))) { console.log(`Matched number: ${match[0]}`); console.log(`Starting index: ${match.index}`); console.log(`Next search will start at index ${regex.lastIndex}`); } code.innerHTML = `const regex = /(\d+)/g; <br> const str = '2022 is almost over, and 2023 is around the corner.'; <br> <br> let match; <br> while ((match = regex.exec(str))) { <br> console.log(\`Matched number: \${match[0]}\`); <br> console.log(\`Starting index: \${match.index}\`); <br> console.log(\`Next search will start at index \${regex.lastIndex}\`); <br> } <br> <br> // Output: <br> // Matched number: 2022 <br> // Starting index: 0 <br> // Next search will start at index 4 <br> // Matched number: 2023 <br> // Starting index: 29 <br> // Next search will start at index 33`; </script> </body> </html>
Output
Conclusion
The lastIndex property in JavaScript regex objects allows us to manage our matches within strings, and give us the index from where to begin our search for a string in consecutive turns. It keeps track of the index where the next search will start and allows us to perform consecutive searches efficiently. We learned the concept of lastIndex in JavaScript regex matching and saw some examples explaining the same.