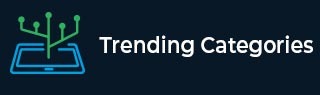
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
The best way to remove duplicates from an array of objects in JavaScript?
Let’s say the following is our array of objects with duplicates −
var studentDetails=[ {studentId:101}, {studentId:104}, {studentId:106}, {studentId:104}, {studentId:110}, {studentId:106}, ]
Use the concept of set to remove duplicates as in the below code −
Example
var studentDetails=[ {studentId:101}, {studentId:104}, {studentId:106}, {studentId:104}, {studentId:110}, {studentId:106}, ] const distinctValues = new Set const withoutDuplicate = [] for (const tempObj of studentDetails) { if (!distinctValues.has(tempObj.studentId)) { distinctValues.add(tempObj.studentId) withoutDuplicate.push(tempObj) } } console.log(withoutDuplicate);
To run the above program, you need to use the following command −
node fileName.js.
Output
Here, my file name is demo158.js. This will produce the following output −
PS C:\Users\Amit\JavaScript-code> node demo158.js [ { studentId: 101 }, { studentId: 104 }, { studentId: 106 }, { studentId: 110 } ]
Advertisements