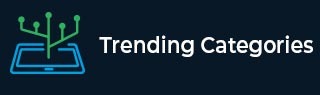
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Switch statements in Dart Programming
Switch statements help us in cases where we want to run specific code based on certain conditions. It's true that if-else conditions also help us in the same section of code, but the switch statements reduce the complexity of the program as we will end up with less code in case of a switch if the condition checks are dense.
Syntax
switch(case){ case x: // do something; break; case y: // do something; break; default: // do something; }
Example
Consider the example shown below −
void main() { var name = "rahul"; switch(name){ case "mukul": print("it is mukul"); break; case "rahul": print("it is rahul"); break; default: print("sorry ! default case"); } }
The parentheses that are followed by the switch keyword contains the variable we want to match with the different cases we have inside the switch code block, when it matches a specific case, the statement that is written inside the code block of that case will be executed and the code will exit from the switch case, as we have a break statement there.
Output
it is rahul
It should be noted that it is necessary to have the break keyword in every switch case, as the compiler will through an error if we don't have one.
Example
Consider the example shown below −
void main() { var name = "rahul"; switch(name){ case "mukul": print("it is mukul"); case "rahul": print("it is rahul"); break; default: print("sorry ! default case"); } }
Output
Error: Switch case may fall through to the next case. case "mukul": ^ Error: Compilation failed.
In most of the languages, we put break statements so that we can fallthrough to the next case. We can achieve that scenario in dart also.
Example
Consider the example shown below −
void main() { var piece = 'knight'; switch(piece) { case 'knight': case 'bishop': print('diagonal'); break; } }
Output
diagonal