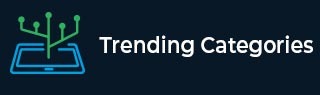
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Swift Program to Split a set into Two Halves
In Swift, a set is used to create an unordered collection of unique elements. To split a set into two halves we use a approach in which we first determine the midpoint of the given set using integer division method and then loop through the set and insert element into either the first half or second half set according to the index related to the midpoint.
For example −
Original Set - [2, 4, 5, 6, 7, 1] Set1 - [2, 4, 5] Set2 - [6, 7, 1]
Algorithm
Step 1 − Create a function which takes a set as a parameter and then split the set into two halves and return the result in a tuple.
Step 2 − Inside the function, find the size of the given set.
Step 3 − Find the midpoint of the given set using integer division method.
Step 4 − Create two new sets named as firstHalfSet and secondHalfSet to store first half and second half of the given set.
Step 5 − Now run a for-in loop to insert data into firstHalfSet and secondHalfSet according to the midpoint.
Step 6 − Return a tuple which contains firstHalfSet and secondHalfSet.
Step 7 − Now outside the function create a set of integer type.
Step 8 − Call the above created function and pass the set as a parameter.
Step 9 − Print the output.
Example
In the following example, we will create a user defined function named as splitSet(). Which takes a set as a parameter and return a tuple of two sets. Inside the function, first we find the size of the set using count property and then find the mid-point by dividing the size of set by 2. Then we create two sets to store the first half and the second half of the set. Then run a for-in loop to iterate through each element of set and check if the current index is less than the midpoint. If yes, then enter the element present at the currentIndex into firstHalfSet. Otherwise, enter the element into secondHalfSet. And in each iteration the value of current index is increased by one, this process continue till the end of the given set. And then return a tuple which contain two sets. Outside the function we create a set of integer type: [99, 22, 33, 66, 11, 55] and pass it into the splitSet() and then store the result into a tuple and then display the two halves of the given set.
import Foundation import Glibc // Function to divide the set into two halves func splitSet(myset: Set<Int>) -> (Set<Int>, Set<Int>) { let setSize = myset.count // Midpoint of set let setMidpoint = setSize / 2 // Creating new sets for storing data var firstHalfSet: Set<Int> = [] var secondHalfSet: Set<Int> = [] var currentIndex = 0 // Run for-in loop to insert data into new sets according to the midpoint for number in myset { if currentIndex < setMidpoint { firstHalfSet.insert(number) } else { secondHalfSet.insert(number) } currentIndex += 1 } return (firstHalfSet, secondHalfSet) } // Test case let mset: Set<Int> = [22, 99, 33, 66, 11, 55, 33] let (Set1, Set2) = splitSet(myset: mset) print("Original set is:", mset) print("First half of the given set:", Set1) print("Second half of the given set:", Set2)
Output
Original set is: [11, 66, 22, 99, 55, 33] First half of the given set: [11, 66, 22] Second half of the given set: [99, 33, 55]
Conclusion
So this is how we can split a set into two halves. However it is not necessary that the order of the elements in the resultant sets are same as the original set because sets are unordered collections.