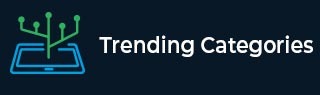
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Swift Program to Show Overloading of Methods in Class
Method overloading is a technique that allows us to create multiple methods of same name within a single class but with different parameter types or different number of parameters. The specific method that is called is determined by the types or number of arguments passed to it at the time of the method call. For example −
class Book { // Methods func Author(){} func Author(x: Int)->Int{} func Author(x: String)->String{} func Author(a: Int, b: String)->Int{} }
Here, the class Book has four overloaded methods. Where the names of the methods are the same but the parameter type and the number of parameters are different. In this article, we will learn how to write a swift program to show the overloading of methods in the class.
Method 1: Overloading with Different Number of Parameters
Here a class contain multiple methods of same name but with different number of parameters.
Example
Following Swift program to show method overloading in a class with different number of parameters.
import Foundation import Glibc class MathematicOperation { // Method 1 func product(x: Int, y: Int) -> Int { return x * y } // Method 2 func product(x: Int, y: Int, z: Int) -> Int { return x * y * z } } // Creating object let obj = MathematicOperation () // Calling method 1 print("Product:", obj.product(x: 10, y: 40)) // Calling method 2 print("Product:", obj.product(x: 80, y: 20, z: 90))
Output
Product: 400 Product: 144000
Here in the above code, we create a class named “MathematicOperation". This class contain two methods with same name that is “product”, but with different number of parameters. Then we create an instance of the “MathematicOperation” class and call the “product” method. We can determine which method is called according to their number of parameters. If we pass two parameters, then the first method is called whereas if we pass three parameters then the second method is called.
Method 2: Overloading with Different Type of Parameters
Here a class contain multiple methods of same name but with different type of parameters. Here the number of parameters can be same but their type must be different.
Example
Following Swift program to show method overloading in a class with different type of parameters.
import Foundation import Glibc class Addition { // Method 1 func sum(x: Int, y: Int) -> Int { return x + y } // Method 2 func sum(x: Double, y: Double) -> Double { return x + y } // Method 3 func sum(x: String, y: String)-> String{ return x + y } } // Creating object let obj = Addition() // Calling method 1 print("Sum:", obj.sum(x: 10, y: 40)) // Calling method 3 print("Sum:", obj.sum(x: "Blue", y: "Sky")) // Calling method 2 print("Sum:", obj.sum(x: 82.2, y: 21.2))
Output
Sum: 50 Sum: BlueSky Sum: 103.4
Here in the above code, we create a class named “Addition". This class contains three methods with the same name that is “sum” and the same number of parameters but with a different type of parameters. Then we create an instance of the “Addition” class and call the “sum” methods. We can determine which method is called according to their type of parameters. If we pass two parameters of integer type, then the first method is called. If we pass two parameters of string type, then the third method is called. If we pass two parameters of double type, then the second method is called.
Conclusion
Therefore, this is how we can show the overloading of methods in the class. Method overloading provides better code readability, flexibility, simplicity, and polymorphism. In addition, always remember the overloaded methods can have the same name, the same or different return types, but their parameter list should be different.