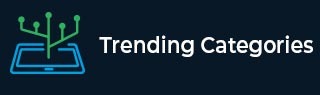
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Swift Program to Remove an Element from the Set
This tutorial will discuss how to write swift program to remove an element from the set.
Set is a primary collection type in Swift. It is an unordered collection which stores unique values of same data type. You are not allowed to store different type of values in the same set. A set can be mutable or immutable.
To remove an element from the set Swift provide an in-built library function named remove(). The remove() function delete the given element from the specified set. If the given element is not the part of the specified set, then it will return nil.
Below is a demonstration of the same −
Input
Suppose our given input is −
MySet = [23, 45, 67, 8, 90, 10, 100] Remove = 45
Output
The desired output would be −
Modified Set = [23, 67, 8, 90, 10, 100]
Syntax
Following is the syntax −
setName.remove()
Algorithm
Following is the algorithm −
Step 1− Create a set with values.
Step 2− Remove an element from the set using remove() function.
Step 3− Print the output
Example 1
The following program shows how to remove an element from the set.
import Foundation import Glibc // Creating a set of integer type var setNumbers : Set = [44, 100, 10, 19, 29, 200, 300, 34] print("Original Set:", setNumbers) // Remove element from the set setNumbers.remove(10) print("Modified Set:", setNumbers)
Output
Original Set: [100, 34, 29, 300, 200, 44, 10, 19] Modified Set: [100, 34, 29, 300, 200, 44, 19]
Here, in the above code, we have a set of integer type named setNumbers. Now we remove an element that is 10 from the set using remove () function −
setNumbers.remove(10)
Hence after removing element the resultant set is [100, 34, 29, 300, 200, 44, 19]
Example 2
The following program shows how to remove an element from the set.
import Foundation import Glibc // Creating a set of string type var setNames : Set = ["Hen", "Owl", "Duck", "Eagle", "Bee"] print("Original Set:", setNames) // Element var newEle1 = "Owl" var newEle2 = "Parrot" // Remove element from the set // Here the given element is the part of the set if let res1 = setNames.remove(newEle1){ print("Removed Element 1: ", res1) } // Remove element from the set // Here the given element is not the part of the set if let res2 = setNames.remove(newEle2){ print("Removed Element 1: ", res2) } else{ print("Removed Element 2: Nil") } print("Modified Set:", setNames)
Output
Original Set: ["Bee", "Eagle", "Hen", "Duck", "Owl"] Removed Element 1: Owl Removed Element 2: Nil Modified Set: ["Bee", "Eagle", "Hen", “Duck”]
Here, in the above code, we have a set of String type named setNames. Now we remove two elements that are “owl” and “parrot” from the set using remove() function. When element is “owl” then it will remove “owl” from the set and return the removed element in optional type. Whereas when we remove “parrot” from the set, then it will return nil because parrot is not the part of setNames set. Here, the remove() function return element in optional type, so we use ! Or let to unwrap optional type. Hence the final set is ["Bee", "Eagle", "Hen", “Duck”].