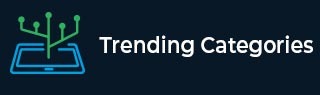
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Swift Program to print the right diagonal matrix
A matrix is an arrangement of numbers in rows and columns. Matrix can be of various type like square matrix, horizontal matrix, vertical matrix etc. So here we print the right diagonal of square matrix. Square matrix is a matrix in which the number of rows and columns are same. For example 3x3, 5x5, 7x7, etc.
In this article, we will learn how to write a swift program to print the right diagonal matrix.
Algorithm
Step 1 − Create a function.
Step 2 − Run for-in loop to iterate through each element of the matrix.
Step 3 − Check for the right diagonal elements that is ((x+y)==(S-1)).
Step 4 − If the element are right diagonal elements, then print 3. Otherwise. Print 0.
Step 5 − Call the function and pass the matrix size to it.
Example
Following Swift program to print the right diagonal matrix.
import Foundation import Glibc // Function to print the right diagonal matrix func printRightDiagonal(S: Int) { for x in 0..<S { for y in 0..<S { if ((x+y) == (S-1)) { print("3", terminator: " ") } else { print("0", terminator: " ") } } print() } } // Calling the function and passing // the size of the square matrix printRightDiagonal(S: 6)
Output
0 0 0 0 0 3 0 0 0 0 3 0 0 0 0 3 0 0 0 0 3 0 0 0 0 3 0 0 0 0 3 0 0 0 0 0
Conclusion
Here in the above code, we create a function to print the right diagonal square matrix. As we know the that the size of rows and columns are same, so in our case the size is 6, means number of rows = 6 and number of columns = 6. So in this function, we use nested for-in loop which iterates through each row and column. Then check if the row and column indices are same that is for the right diagonal elements((x+y)==(S-1)). If yes then this function print 3. Otherwise print 0. So this is how we can print the right diagonal matrix. Here this method works only for square matrix not for other matrix like 4x5, 6x8, etc.