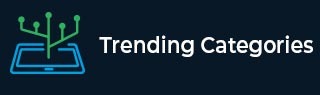
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Swift Program to find the tangent of given radian value
This tutorial will discuss how to write a Swift program to find the tangent of given radian value.
A tangent function is used to define the ratio of the length of the opposite side to the adjacent side in the right-angled triangle. It is also known as the tan function. The mathematical representation of the tangent() function is −
tan() = opposite side/adjacent Side
In Swift, we can calculate the tangent of the given radian value using the pre-defined tan() function. This function returns the tangent value of the specified number. Here, the specified number represent an angle.
Syntax
Following is the syntax −
tan(Num)
Here, the value of Num can be of integer, float, or double type.
If the given value is in degrees then we can convert degrees to radians using the following formula −
Radians = Degrees * (pi / 180)
Below is a demonstration of the same −
Input
Suppose our given input is
Number = 2.1
Output
The desired output would be
The value of tan 2.1 is 1.7098465429045073
Algorithm
Following is the algorithm
Step 1 − Import Foundation library to use mathema tic functions. import Foundation
Step 2 − Declare variable to store radian value.
Step 3 − If the value is in degrees then use the following formula −
If the value is in radian the ignore this step.
Step 4 − Find the tangent value using the tan() function −
var res1 = tan(tNum1)
Step 5 − Print the output.
Example 1
Finding tangent of given radian value
The following program shows how to find the tangent of the given radian value.
import Foundation import Glibc var tNum1 : Double = 1.3 var tNum2 : Double = 1.3 // Calculating the cosine of the radian value // Using tan() function // For positive radian value var res1 = tan(tNum1) // For negative radian value var res2 = tan(tNum2) print("The value of tan\(tNum1) is ",res1) print("The value of tan \(tNum2) is ", res2)
Output
The value of tan 1.3 is 3.6021024479679786 The value of tan 1.3 is 3.6021024479679786
Here, in the above code, we find the tangent value of the given radian using the tan() function −
var res1 = tan(tNum1) var res2 = tan(tNum2)
Display the result: tan 1.3 is 3.6021024479679786 and tan -1.3 is -3.6021024479679786.
Example 2
Finding tangent of given degrees value
The following program shows how to find the tangent value of the given degrees.
import Foundation import Glibc var tNum1 = 60.0 var tNum2 = 30.0 // Convert degrees into radian var radian1 = tNum1 * (Double.pi / 180) var radian2 = tNum2 * (Double.pi / 180) // Calculating the tangent value // Using tan() function var res1 = tan(radian1) var res2 = tan(radian2) print("The value of tan\(tNum1) degrees is ",res1) print("The value of tan\(tNum2) degrees is ",res2)
Output
The value of tan60.0 degrees is 1.7320508075688767 The value of tan30.0 degrees is 0.5773502691896257
Here, in the above code, we calculate the value of tangent of the given degrees. Here, first we convert the degrees into radian using the following code −
var radian1 = tNum1 * (Double.pi / 180)
And then calculate the tangent value using the tan() function −
var res1 = tan(radian1)
Display the result: tan 60.0 degrees is 1.7320508075688767 and tan 30.0 degrees is 0.5773502691896257