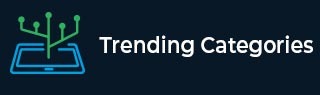
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Swift Program to Find the Frequency of Characters in a String
In Swift, the frequency of all the characters in a string means how much time a character repeats in the given string. For example, “Swift tutorial”, here “t” repeats 3 times in the given string, “i” repeat 2 times in the given string. So in this article, we are going to find the frequency of all the characters in the string.
Algorithm
Step 1 − Create a variable to store a string.
Step 2 − Create a dictionary to store the character and its count.
Step 3 − Run a for loop to iterate over each character in the input string.
Step 4 − Now we check if the current character is present more than 1 time or not, If yes, then increase the size by 1 on each occurrence.
Step 5 − If not, then store the current character in the dictionary with size 1.
Step 6 − Again run a for-in loop to display the character and its total occurrence in the string.
Example
In the following Swift program, we will find the frequency of characters in a string. So first we will create a string, and an empty dictionary to store the characters and their frequency count. Then we will run a for-in loop to iterate through each character of the input string. If a character occurs more than one time then, we will increase its count by one on each occurrence. If not, then we assign we set its count value to one. And finally, we will display the results on the output screen.
import Foundation import Glibc let EnteredString = "This is Swift Tutorial" print("Input String is: ", EnteredString) var charCount = [Character:Int]() for C in EnteredString { if let size = charCount[C]{ charCount[C] = size+1 } else { charCount[C] = 1 } } for (C, size) in charCount { print("Character:\(C) occurs \(size) times in the given string") }
Output
Input String is: This is Swift Tutorial Character:S occurs 1 times in the given string Character:a occurs 1 times in the given string Character:l occurs 1 times in the given string Character:w occurs 1 times in the given string Character:i occurs 4 times in the given string Character:T occurs 2 times in the given string Character:o occurs 1 times in the given string Character:u occurs 1 times in the given string Character:t occurs 2 times in the given string Character:f occurs 1 times in the given string Character:r occurs 1 times in the given string Character:s occurs 2 times in the given string Character:h occurs 1 times in the given string Character: occurs 3 times in the given string
Conclusion
So this is how we can find the frequency or the occurrence of all the characters present in the given string. The above-discussed method finds the total count of the occurrence of all the characters along with the total white spaces used in the given string. So with the minor changes in the code, you can only find the total white space used in the given string.