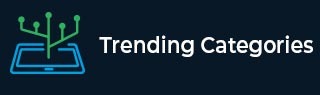
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Swift Program to fill an array with a specific element
In this article, we will learn how to write a swift program to fill an array with a specific element.
An array is used to store elements of same data type in an order whereas a set is used to store distinct elements of same data type without any definite order. In an array, every element has an index. The array index is start from 0 and goes up to N-1. Here N represent the total number of array elements.
We can fill an array with a specified element using the following methods −
Using user defined function
Using array initialisation
Using map() function
Using indices property
Method 1: Using user-defined Function
To fill the given array with a specified element we create a function in which we run a for loop to iterate through each element of the given array and assign the specified element to each element of the given array.
Example
Following Swift program to fill an array with a specific element.
import Foundation import Glibc // Function to fill an array with the specified element func fillArray<T>(arr: inout [T], with element: T) { for i in 0..<arr.count { arr[i] = element } } var arr = [34, 5, 7, 78, 2, 11, 67, 8] print("Original Array:", arr) fillArray(arr: &arr, with: 1) print("Modified Array:", arr)
Output
Original Array: [34, 5, 7, 78, 2, 11, 67, 8] Modified Array: [1, 1, 1, 1, 1, 1, 1, 1]
Here in the above code, we have an array of integer type. Now we create a function to fill the array with the specified element that is 1. So in this function, we run a for loop to iterate through each element of the given array and assign the element with the specified value that is 1.
Method 2: Using Array Initializer
We can also use array initializer to create an array the is filled with a specified element.
Syntax
Array(repeating: Value, Count:Value)
Here, repeating parameter specifies the element to be repeated and the count parameter specifies the number of the times element should be repeated.
Example
Following Swift program to fill an array with a specific element.
import Foundation import Glibc let ele = "Pink" let countVal = 6 // Creating and filling array let mArray = Array(repeating: ele, count: countVal) print("Array is:", mArray)
Output
Array is: ["Pink", "Pink", "Pink", "Pink", "Pink", "Pink"]
Here in the above code, we create an array using Array() initializer and inside this Array initialiser we pass the value of repeating and count parameter. Now this array() initializer fill the given array with “Pink” element and repeat this element 6 times.
Method 3: Using map(_:) function
We can also fill an array with a specified element using map(_:) function. The map(_:) function is used to return an array containing the results of mapping the given closure over the array’s elements.
Syntax
func map<T>(_t:(Self.Element) throws-> T)rethrows- >[T]
Here, t is a mapping closure. It takes an element of the given array as its parameter and return a transformed value of the same or different type.
Example
Following Swift program to fill an array with a specific element.
import Foundation import Glibc // Declare an array with 9 elements var myArr = [3, 5, 6, 7, 3, 3, 2, 3, 2] print("Original Array:", myArr) // Fill the array with the value 9 myArr = myArr.map { _ in 9 } // Print the modified array print("Modified Array:", myArr)
Output
Original Array: [3, 5, 6, 7, 3, 3, 2, 3, 2] Modified Array: [9, 9, 9, 9, 9, 9, 9, 9, 9]
Here in the above code, we create an array of integer type. Now using map() function we fill the given array elements with 9. So to do this we pass a closure { _ in 9 } in the map() function and then map() function will fill each element of the given array with element 9.
Method 4: Using Indices Property
To fill the given array with a specified element we can use indices property of the array.
Example
Following Swift program to fill an array with a specific element.
import Foundation import Glibc // Declare an array with 5 elements var number = [Int](repeating: 0, count: 5) let element = 10 // Fill the array with element 10 for x in number.indices { number[x] = element } print("Array:", number)
Output
Array: [10, 10, 10, 10, 10]
Here in the above code, we declare an array with 5 element. Now we run a for loops to iterate over the indices property of the array and assign the value of the element = 10 variable to each index.
Conclusion
Therefore, this is how we can fill an array with a specified element. Here, always remember the element type is equal to the array type.