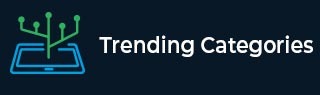
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Swift Program to Display All Prime Numbers from 1 to N
This tutorial will discuss how to write a Swift program to display all prime numbers from 1 to N.
Prime numbers are those numbers that are greater than 1 and has exactly two factors that is 1 and the number itself. For example, 2 is the prime number because it has only two factors that are 1 and 2.
Below is a demonstration of the same −
Suppose we enter the following input −
Range - 1 to 20
Following is the desired output −
Prime numbers between 1 to 20 are - 2, 3, 5, 7, 11, 13, 17, 19
Algorithm
Following is The algorithm to display all prime numbers from 1 to N −
Step 1 − Create a function which will return the prime numbers named as “checkingPrimeNumber()”.
Step 2 − Check if the given number is 1 or 0 using if statement. If the given number is 1 or 0 then return false. Which means the number is not prime number.
Step 3 − Run a for loop from 2 to num-1
Step 4 − Inside for loop check if the given number is divisible by j. If the number is divisible by j then return false, that means the number is not prime number otherwise the number is prime number so return true.
Step 5 − Create two variables that store lower and upper limit. Here the value of these variable can be pre-defined or user-defined.
Step 6 − Run another for loop from lower to upper limit.
Step 7 − Display all the prime numbers present in between the given range by calling the checkingPrimeNumber().
Example 1
The following program shows how to display all prime numbers from 1 to N.
import Foundation import Glibc func checkingPrimeNumber(num: Int) -> Bool{ // Checking if num for 1 or 0 // 1 and 0 are not prime number. // So, if num is 1 or 0, then return false // Which means the number is not prime number if(num == 1 || num == 0){ return false } // For loop starts from 2 to num - 1 for j in 2..<num{ // Checking the num is divisible by j, if yes // then the num is not prime number if (num % j == 0){ return false } } // Else num is prime number return true } var lowerLimit = 1 var upperLimit = 20 print("Range-\(lowerLimit) to \(upperLimit)") print("Prime numbers are :") // Checking for every number staring from // LowerLimit to upperLimit(that is 1 to 20) for k in lowerLimit...upperLimit{ // Checking if the current number is // prime number or not if (checkingPrimeNumber(num: k)){ // Display only prime number print(k) } }
Output
Range-1 to 20 Prime numbers are : 2 3 5 7 11 13 17 19
Here, in the above code we create a function named as checkingPrimeNumber(), this function takes one argument and return the prime number. Now we declare two variables with values named as lowerLimit = 1 and upperLimit = 20. We iterate a for loop from 1 to 20 and display all the prime numbers present in between 1 to 20 by calling the checkingPrimeNumber() function.
Example 2
The following program shows how to display all prime numbers from 1 to N by taking user input.
import Foundation import Glibc func checkingPrimeNumber(num: Int) -> Bool{ // Checking num for 1 or 0 // because 1 and 0 are not prime number. if(num == 1 || num == 0){ return false } // For loop starts from 2 to num - 1 for j in 2..<num{ // Checking the num is divisible by j, if yes // then the num is not prime number if(num % j == 0){ return false } } // Else the number is prime number return true } print("Please enter lower range:") // Enter the integer type lower range from the user var lowerLimit = Int(readLine()!)! print(lowerLimit) print("Please enter upper range:") // Enter the integer type upper range from the user var upperLimit = Int(readLine()!)! print(upperLimit) print("Entered range is \(lowerLimit) to \(upperLimit)") print("So following are the Prime numbers:") // Checking for every number staring from // LowerLimit to upperLimit for k in lowerLimit...upperLimit{ // Checking if the current number is // prime number or not if (checkingPrimeNumber(num: k)){ // Display only prime numbers print(k) } }
STDIN Input
Please enter lower range: 1 Please enter upper range: 30
Output
So following are the Prime numbers: 2 3 5 7 11 13 17 19 23 29
Here, in the above code we take the value of lower limit and upper limit from the user and display all the prime numbers in between the specified range by calling checkingPrimeNumber() function.