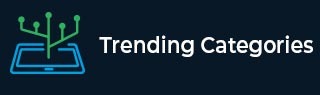
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Swift Program to convert the string into an array of characters based on the specified character
In Swift, convert the string into an array of characters based on the specified character using the split() function. The split() function splits the given string at the specified separator and returns the result in an array.
Input
String = “Today is cloudy day” Character = “y”
Output
[“toda”, “is cloud”, “da”]
Here, the string is split at character “y” and converted the split string into an array.
Syntax
func.split(separator:Character, maxSplits Int, ommittingEmptySequence:Bool)
The split function takes the following arguments −
separator − It is an element at which the split operation happens.
maxSplits − It is an optional parameter. It is used to set the maximum number of splits. The default value of this parameter is set to Int.max.
omittingEmptySeubsequences − It is also an optional parameter. It is used to remove empty string elements from the resultant array. By default, it is set to true.
Algorithm
Step 1 − Declare a variable to store a string.
Step 2 − Declare a variable to a character at which we want to split the string.
Step 3 − Now split the string using the split() function and pass the character as a value of the separator argument. And store the result in a variable.
Step 4 − Display the output.
Example
The following Swift program will convert the string into an array based on the specified character. So create a string and a separator. Then use the split() function to split the string according to the specified character that is “I” and store the result into a variable. And display the resultant array.
import Foundation import Glibc let InputStr = "MyI caIr is pInk" // Character to separate string let sChar: Character = "I" // Convert the string into an array of characters // according to the given separator let CharArr = InputStr.split(separator: sChar) print("Array:", CharArr)
Output
Array: ["My", " ca", "r is p", "nk"]
Conclusion
So this is how we can convert the string into an array of characters based on the specified character using the split() function. This function converts the given string into an array where each string is separated at the specified character. Here you can use any separator according to your choice. Also, the time complexity of the split() function is O(x) where x represents the length of the given string.