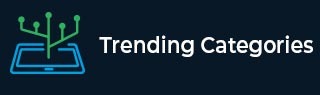
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Super Ugly Numbers JavaScript
Super Ugly Number
Super ugly numbers are positive numbers whose all prime factors are in the given prime list primes of size k. For example, [1, 2, 4, 7, 8, 13, 14, 16, 19, 26, 28, 32] is the sequence of the first 12 super ugly numbers given primes = [2, 7, 13, 19] of size 4.
Problem
We are required to write a JavaScript function that takes in number, num, as the first argument and an array, arr, of prime numbers as the second argument. The function should find and return the (num)th super ugly number.
Example
The code for this will be −
const num = 7; const arr = [2, 7, 14, 19]; const superUgly = (num = 1, arr = []) => { arr.sort((a, b)=> a - b); const ptr = []; const res = []; for(let i=0;i<arr.length;i++){ ptr[i] = 0; }; res.push(1); for(let i = 1; i < num; i++){ let mn=Math.pow(2, 32) - 1; for(let j = 0; j < arr.length; j++){ mn=Math.min(mn,arr[j]*res[ptr[j]]) }; res[i]=mn for(let j=0; j < arr.length; j++){ if(mn % arr[j] === 0){ ptr[j]++; }; }; }; return res[num-1] }; console.log(superUgly(num, arr));
Output
And the output in the console will be −
16
Advertisements