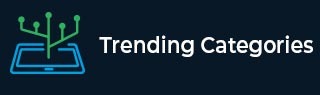
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Sum of even numbers from n to m regardless if nm JavaScript
We are required to write a function that takes two numbers as arguments m and n, and it returns the sum of all even numbers that falls between m and n (both inclusive)
For example −
If m = 10 and n = -4
The output should be 10+8+6+4+2+0+(-2)+(-4) = 24
Approach
We will first calculate the sum of all even numbers up to n and the sum of all even numbers up to m.
Then we will check for the bigger of the two m and n. Subtract the sum of smaller from the sum of bigger which will eventually give us the sum between m and n.
Formula
Sum of all even number from 0 to N is given by −
$$\frac{N\times(N+2)}{4}$$
Therefore, let’s put all this into code −
Example
const sumEven = n => (n*(n+2))/4; const evenSumBetween = (a, b) => { return a > b ? sumEven(a) - sumEven(b) + b : sumEven(b) - sumEven(a) + a; }; console.log(evenSumBetween(-4, 10)); console.log(evenSumBetween(4, 16)); console.log(evenSumBetween(0, 10)); console.log(evenSumBetween(8, 8)); console.log(evenSumBetween(-4, 4));
Output
The output in the console will be −
24 70 30 8 0
Advertisements