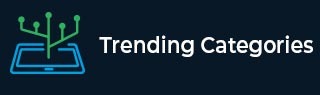
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Sum of array using pointer arithmetic in C
In this program, we need to find sum of array elements using pointer arithmetic.
Here we use * which denotes the value stored at the memory address and this address will remain stored in the variable. Thus “int *ptr” means, ptr is a variable which contains an address and content of the address is an integer quantity.
*p means it is a pointer variable. Using this and sum() we will find out sum of the elements of array.
Example Code
#include <stdio.h> void s(int* a, int len) { int i, s_of_arr = 0; for (i = 0; i < len; i++) s_of_arr = s_of_arr + *(a + i); printf( "sum of array is = %d" ,s_of_arr); } int main() { int arr[] = { 1,2,4,6,7,-5,-3 }; s(arr, 7); return 0; }
Output
Sum of array = 12
Algorithm
Begin Initialize array to hold the variables. Call function s to get the sum of the variables. Print the sum. End.
Advertisements