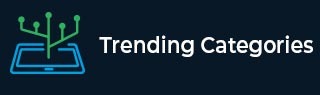
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
StringJoiner length() method in Java 8
The length() method of the StringJoiner class in Java 8 is used to get the length of the current value of StringJoiner.
To work with the StringJoiner in Java 8, import the following package:
import java.util.StringJoiner;
The syntax is as follows:
public int length()
First, create a StringJoiner:
StringJoiner strJoin = new StringJoiner(","); strJoin.add("US"); strJoin.add("India"); strJoin.add("Netherlands"); strJoin.add("Australia");
Now, find the length of the StringJoiner i.e. the number of elements in it using the length() method:
strJoin.length());
The following is an example to implement StringJoiner length() method in Java:
Example
import java.util.StringJoiner; public class Demo { public static void main(String[] args) { // StringJoiner StringJoiner strJoin = new StringJoiner(","); strJoin.add("US"); strJoin.add("India"); strJoin.add("Netherlands"); strJoin.add("Australia"); System.out.println("Length of the StringJoiner = "+strJoin.length()); System.out.println("StringJoiner = "+strJoin.toString()); } }
Output
Length of the StringJoiner = 30 StringJoiner = US,India,Netherlands,Australia
Advertisements