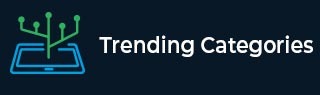
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
String Without AAA or BBB in C++
Suppose we have two integers A and B, we have to return any string S, such that −
S has length A + B and contains exactly A number of letter ‘a’ and B number of ‘b’ letters.
Substring “aaa” and “bbb” will not be in the string S
So if the given integers are A = 4, B = 1, then the result will be “aabaa”.
To solve this, we will follow these steps −
Define a string ret, initially this is empty
while |A – B| >= 2,
if A > B, then
ret := ret concatenate ‘aa’
decrease A by 2
if B is non-zero concatenate ‘b’ with ret and decrease B by 1
else
ret := ret concatenate ‘bb’
decrease B by 2
if A is non-zero concatenate ‘a’ with ret and decrease A by 1
while either A is non-zero, or B is non-zero
if A is non zero and (size of ret < 2 or not of (size of ret >= 2 and last element of ret = second last element of ret) and last element of ret is ‘a’), then
ret := ret + ‘a’, decrease A by 1
if B is non-zero
ret := ret concatenate ‘b’, decrease B by 1
otherwise ret concatenate ‘b’, decrease B by 1, if A is not 0, then concatenate a with ret and decrease A by 1
return ret
Let us see the following implementation to get better understanding −
Example
#include <bits/stdc++.h> using namespace std; class Solution { public: string strWithout3a3b(int A, int B) { string ret = ""; while(abs(A - B) >= 2){ if(A > B){ ret += 'a'; ret += 'a'; A -= 2; if(B) { ret += 'b'; B--; } }else{ ret += 'b'; ret += 'b'; B -= 2; if(A) { ret += 'a'; A--; } } } while(A || B){ if(A && (ret.size() < 2 || !(ret.size() >= 2 && ret[ret.size() - 1] == ret[ret.size() - 2] && ret[ret.size() - 1] == 'a') ) ){ ret += 'a'; A--; if(B) { ret += 'b'; B--; } }else{ ret += 'b'; B--; if(A) { ret += 'a'; A--; } } } return ret; } }; main(){ Solution ob; cout << (ob.strWithout3a3b(4, 1)); }
Input
4 1
Output
"aabaa"