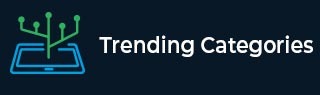
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
String slicing in Python to check if a can become empty by recursive deletion
In this tutorial, we are going to write a program that will check whether the given string can become empty or not by recursive deletion of chars using slice. Let's see an example to understand it more clearly.
Input
string = "tutorialstutorialspointpoint" sub_string = "tutorialspoint"
Output
True
- After first iteration tutorialstutorialspointpoint becomes tutorialspoint.
- After the second iteration, the string will be empty.
We can achieve the result using find() method of the string. Follow the below steps to write the program.
- Initialize the string and sub_string.
- If any of them is empty, then return False
- While string length is greater than zero. Do the following.
- Check whether the sub_string is present in string or not.
- If not present, then return False
- Return True as the loop doesn't terminate in middle.
Example
def is_valid(string, sub_string): # checking the lengths of string and sub_string if len(string) > 0 and len(sub_string): # iterating until string becomes empty while len(string) > 0: # finding the sub_string in string index = string.find(sub_string) # checking whether its present or not if index == -1: # returning false return False # removind the sub_string string = string[0: index] + string[index + len(sub_string):] # returning True return True else: # returning False return False if __name__ == '__main__': # initializing the string and string string = 'tutorialstutorialspointpoint' sub_string = 'tutorialspoint' # invoking the method print(is_valid(string, sub_string))
Output
If you run the above code, then you will get the following result.
True
Conclusion
If you have any queries in the tutorial, mention them in the comment section.
Advertisements