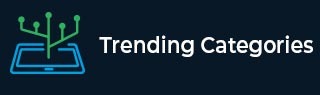
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Sorting an integer without using string methods and without using arrays in JavaScript
In the given problem statement, our task is to sort an integer number without the usage of string methods and without usage of arrays with the help of Javascript functionalities. So for solving this task we will use mathematical operations and loops in Javascript.
Understanding the Problem
The problem says to sort the given integer number without using the string and array method in Javascript. The question arises about sorting the integer that means we have to array its digits in ascending or descending order.
For example suppose we have an integer number as 784521, so the sorted result will be 124578 in ascending order and 875421 in descending order. So basically our task is to perform the technique to give the same result without including string and array methods. So we will employ a mathematical approach and extract every digit from the given number and insert it into the correct place in the sorted number.
Logic for the given Problem
To sort the given integer we will basically create a function to do this task. And the function accepts an integer as input and will sort its digits in descending order. And we will also use a helper function to insert every digit of the number at the correct place in the sorted number. The procedure will iterate over the digits of the original number and compare them with the current digit which is being inserted. And lastly we will have the sorted number in results.
Algorithm
Step 1: Declare a function and give it a name as sortInteger and the task of this function is to sort the given input integer.
Step 2: Define a variable to store the sorted integers and give it name as sortedNum and initialize it with zero.
Step 3: Now use a while loop and run this loop until the given number becomes zero. Inside the loop we will extract the last digit of the number after calculating the remainder of the number divided by 10. And assign the result to a variable digit. Call the helper function and give it a name as insertDigit and inside the function we will pass sortedNum and digit as parameter. And update the number.
Step 4: Using another while loop we will calculate the current digit and if the value is less than the current digit and inserted is false then we will add digit multiplied by multiplier to the new number and set it to true.
Step 5: If inserted is still false then add a digit multiplied by the multiplier to a new number and return the new number as the result.
Example
//Function to sort the given integer numbers function sortInteger(num) { if (num < 0) { // Negative numbers is not supported return -1; } let sortedNum = 0; while (num > 0) { // Extract the last digit const digit = num % 10; // Insert the digit at the right position sortedNum = insertDigit(sortedNum, digit); num = Math.floor(num / 10); } return sortedNum; } function insertDigit(num, digit) { if (num === 0) { return digit; } let newNum = 0; let multiplier = 1; let inserted = false; while (num > 0) { const currDigit = num % 10; if (digit < currDigit && !inserted) { newNum += digit * multiplier; inserted = true; } newNum += currDigit * (multiplier * (inserted ? 10 : 1)); num = Math.floor(num / 10); multiplier *= 10; } if (!inserted) { newNum += digit * multiplier; } return newNum; } // Testing console.log(sortInteger(302541)); console.log(sortInteger(123456789)); console.log(sortInteger(504030201));
Output
543210 987654321 543210000
Complexity
The function sortInteger iterates over the digits of the given number and it needs O(log n) iterations, here n is the value of input number. And we have also used the insertDigit function which also requires O(log n) iterations in the worst case. Therefore the time complexity of the functions is O(log n * log n). And the space complexity of the create function is O(1) as the code only requires a constant amount of memory to store the variables, so the space does not depend on the size of the input number.
Conclusion
As we have solved the given problem without using string or array manipulation techniques in Javascript. We have just utilized mathematical operations and loops to get and rearrange the digits of the input number. So we have met all the requirements of the given problem.