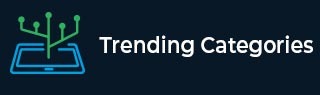
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Sorting a Vector of Numeric Strings in Ascending Order
In this article, we'll examine a C++ procedure for ascendingly sorting an array of numerical string values. Sorting is a basic operation that entails organizing elements in a predetermined order. Because they are character-based strings that represent numbers, and these numerical strings provide a special set of challenges when it is related to sorting. The issue statement, a method and algorithm for solving it, a C++ implementation, a complexity reasoning of the provided approach, and a summary of the main points will all be covered.
Problem Statement
Consider a vector containing numerical strings, the aim is to arrange them in increasing order on the basis of their numerical values. The challenging part is that numerical strings must be arranged according to their numeric values rather than their lexicographic order.
Let's delve into examples to get a better grab at the problem:
Let the Input:["100", "9", "30", "8", "2"]
So, Output: ["2", "8", "9", "30", "100"]
Comment: strings in the input vector represent the integers 100, 9, 30, 8, and 2. The output is ["2", "8", "9", "30", "100"] when these strings are sorted ascendingly, according to their numerical values.
Approach
We use a custom comparator function that deal with both the length and numerical value of the strings to solve the issue of sorting the vector of these strings in an increasing manner. This is how the algorithm works:
Define a comparator function, 'compareNumericStrings', which have two strings as inputs.
Weigh the lengths of the two strings taken above as parameters in the comparator function. 2 cases are possible:
If the lengths are equal:
If the lengths differ:
Use the inbuilt algorithm for sorting, to increasingly organize the strings, specifying compareNumericStrings as the function for comparing the strings.
In this case, we need to perform a lexicographical comparison to figure out their order. The lexicographic comparison considers the ASCII values of the characters to finalize their order.
Example
String1− "11"
String2− "16"
We proceed to the lexicographic comparison because of the same lengths of both strings(2 characters). We can tell that String1's first character is "1" and same with String2's i.e. "1" by analysing the characters from left to right. We now move on to the next(second) character comparison because they are equal. String 1's second character is "1," while String 2's second character is "6". The lexicographical comparison shows that String1 is smaller than String2, since in the ASCII table, '1' appears before '6'.
In this scenerio, the arrangement can be determined directly by comparing the lengths of the strings i.e. one whose length is shorter is considered smaller than the one with a greater length.
Example
String1− "50"
String2− "100"
Here, String1 has a length of 2, while String2 has a length of 3. Clearly, 2 is smaller than 3, therefore, String1 is considered smaller than String2.
Example
#include <iostream> #include <vector> #include <algorithm> using namespace std; // function for sorting numerical strings bool compare_str(const string& str1, const string& str2) { // Lexicographic comparison -> for same-sized strings if (str1.size() == str2.size()) { return str1 < str2; } // Sort by comparing length of strings -> for different-sized strings return str1.size() < str2.size(); } int main() { vector<string> numStrings = { "12", "57", "200", "28", "1" }; // using the comparison function to sort the vector of numeric strings sort(numStrings.begin(), numStrings.end(), compare_str); cout << "Sorted numeric strings in ascending order: "; // printing the sorted numeric strings for (const auto& s: numStrings) { cout << s << " "; } cout << endl; return 0; }
Output
Sorted numeric strings in ascending order: 1 12 28 57 200
Complexity Analysis
Time Complexity− The sorting method used by sort() is the main determinant of the algorithm's time complexity. The sort() function in C++ typically uses the introsort algorithm, which combines the strengths of three different sorting algorithms: quicksort, heapsort, and insertion sort and has average time complexity of O(NlogN). So, O(NlogN) is the time complexity of program, where N is the number of elements in the vector.
Space Complexity− The algorithm only needs a constant amount of space to store the comparison results, making its space complexity O(1).
Explanation Using Test Case
Test case−> {"34", "12", "5", "2"}:
A comparison function called "compare_str" is defined in the code; it accepts two strings as input and returns a boolean value. This function is in charge of comparing two numeric strings based on their lengths and lexicographic order.
The main function begins by invoking the sort function and passing the 'compare_str' comparison function along with the beginning and ending iterators of the numStrings vector. This initiates the action of sorting.
Inside the compare_str function, the comparison logic is as follows:
If the lengths of the two strings being compared are equal, a lexicographic comparison is performed by using the < operator on the two strings (str1 < str2). The result of this comparison is returned.
If the lengths of the strings differ, the comparison is based on the lengths themselves. The function returns the result of comparing the sizes of str1 and str2 using the < operator (str1.size() < str2.size()).
Based on the logic of the comparison function, the sorting operation is performed and the numStrings vector is reorganized in ascending order. The sorted vector in this situation is "2", "5", "12", and "34".
The code in the demonstrated test case arranges the numerical strings in ascending order. The sorted numerical strings "2", "5", "12," and "34" are displayed in the output.
Conclusion
Due to the requirement to take into account both the length and numerical value of the strings, sorting a vector containing numeric strings in ascending order poses a special problem. We can overcome this difficulty by creating a unique comparison function and using the C++ sorting algorithm. A simple answer to this issue is shown by the implementation that is offered.
We have examined the issue statement, presented a strategy and algorithm, offered a C++ implementation, assessed the difficulty of the solution, and emphasized key takeaways in this post. The ability to effectively sort numeric strings is a crucial skill for developers, and the methods described here can be modified to accommodate a variety of sorting cases.