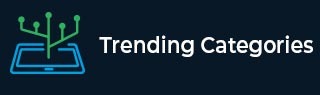
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Sort the matrix row-wise and column-wise using Python
In this article, we will learn a python program to sort the matrix row-wise and column-wise.
Assume we have taken an input MxM matrix. We will now sort the given input matrix row-wise and column-wise using the nested for loop.
Algorithm (Steps)
Following are the Algorithms/steps to be followed to perform the desired task. −
Create a function sortingMatrixByRow() to sort each row of matrix i.e, row-wise by accepting input matrix, m(no of rows) as arguments.
Inside the function, use the for loop to traverse through the rows of a matrix.
Use Another nested for loop to traverse through all the columns of the current row.
Use the if conditional statement to check whether the current element is greater than the next element.
Swap the elements using a temporary variable if the condition is true.
Create another function transposeMatrix() to get the transpose of a matrix by accepting the input matrix, m(no of rows) as arguments.
Use the for loop, to traverse through the rows of a matrix.
Use another nested for loop to traverse through the form (row +1) column to the end of the columns.
Swap the current row, column element with the column, row element.
Create a function sortMatrixRowandColumn() to sort the matrix row and column-wise by accepting the input matrix, m(no of rows) as arguments.
Inside the function, call the above-defined sortingMatrixByRow() function to sort the rows of an input matrix.
Call the above-defined transposeMatrix() function to get the transpose of an input matrix.
Once again sort the rows of an input matrix by calling the above-defined sortingMatrixByRow() function.
Once again getting the transpose of an input matrix by calling the above-defined transposeMatrix() function.
Create a function printingMatrix() to print the matrix by traversing through rows and columns of a matrix using nested for loop.
Create a variable to store the input matrix.
Create another variable to store input m(no of rows) value
Call the above-defined printingMatrix() function to print the input matrix.
Call the above-defined sortMatrixRowandColumn() function by passing the input matrix, m values to it to sort the matrix row and column-wise.
Print the resultant input matrix after sorting row and column-wise by calling the above-defined printingMatrix() function.
Example
The following program returns the sorted matrix by row and column-wise for the given input matrix using nested for loops −
# creating a function for sorting each row of matrix row-wise def sortingMatrixByRow(inputMatrix, m): # traversing till the length of rows of a matrix for p in range(m): # Sorting the current row for q in range(m-1): # checking whether the current element is greater than the next element if inputMatrix[p][q] >inputMatrix[p][q + 1]: # swapping the elements using a temporary variable # if the condition is true tempVariable = inputMatrix[p][q] inputMatrix[p][q] = inputMatrix[p][q + 1] inputMatrix[p][q + 1] = tempVariable # creating a function to get the transpose of a matrix # by accepting the input matrix, m values as arguments def transposeMatrix(inputMatrix, m): # traversing through the rows of a matrix for p in range(m): # Traversing from row +1 column to last column for q in range(p + 1, m): # Swapping the element at index (p,q) with (q,p) temp = inputMatrix[p][q] inputMatrix[p][q] = inputMatrix[q][p] inputMatrix[q][p] = temp # creating a function for sorting the matrix rows column-wise def sortMatrixRowandColumn(inputMatrix, m): # sorting the rows of an input matrix by # calling the above defined sortingMatrixByRow() function sortingMatrixByRow(inputMatrix, m) # getting the transpose of an input matrix by # calling the above defined transposeMatrix() function transposeMatrix(inputMatrix, m) # once again sorting the rows of an input matrix by # calling the above defined sortingMatrixByRow() function sortingMatrixByRow(inputMatrix, m) # once again getting the transpose of an input matrix(So we sorted the columns) transposeMatrix(inputMatrix, m) # creating a function to print the matrix def printingMatrix(inputMatrix, rows): # Traversing in the rows of the input matrix for i in range(rows): # Traversing in the columns corresponding to the current row # of the input matrix for j in range(rows): print(inputMatrix[i][j], end=" ") # Printing a new line to separate the rows print() # input matrix inputMatrix = [[2, 6, 5], [1, 9, 8], [7, 3, 10]] # input m value representing 3x3 matrix # (dimensions) m = 3 print("Input Matrix:") # printing the input matrix by calling the above # printingMatrix() function printingMatrix(inputMatrix, m) # calling the above defined sortMatrixRowandColumn() function # by passing the input matrix, m values to it to # sort the matrix row and column-wise sortMatrixRowandColumn(inputMatrix, m) # printing the input matrix after sorting row and column-wise # by calling the above printingMatrix() function print("Input Matrix after sorting row and column-wise:") printingMatrix(inputMatrix, m)
Output
On execution, the above program will generate the following output −
Input Matrix: 2 6 5 1 9 8 7 3 10 Input Matrix after sorting row and column-wise: 1 5 6 2 7 9 3 8 10
Time Complexity − O(n^2 log2n)
Auxiliary Space − O(1)
Conclusion
In this article, we learned how to use Python to sort the given matrix both row- and column-wise. Additionally, we learned how to transpose the given matrix and how to sort the matrix by rows using nested for loops (rather than utilizing the built-in sort() method).