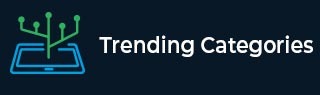
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Simplifying Network Tasks in Python using Paramiko and Netmiko
In today's interconnected world, computer networks play a vital role in facilitating communication and data exchange. As networks grow in complexity, managing and automating network tasks become increasingly important. Python, with its simplicity and versatility, has emerged as a powerful programming language for network automation. In this article, we will explore two popular Python libraries, Paramiko and Netmiko, that simplify network tasks and enable efficient network automation.
Understanding Paramiko
To automate and simplify secure network device communication using the SSH protocol, the Paramiko Python library was developed. For interacting with network hardware, it provides a high−level API that makes it simple to set up SSH connections, execute commands, send files, and handle authentication. The best solution for network automation tasks is Paramiko because of its dependability and simplicity.
To get started with Paramiko, you need to install it using pip:
pip install paramiko
Key Features of Paramiko
SSH Connectivity: You can create SSH connections with network devices using Paramiko, which lets you run commands and send files securely. Both key−based and password−based authentication techniques are supported.
The SSHClient class from Paramiko enables you to connect via SSH to a distant device. During the connection process, you can handle any potential exceptions and set the policy for automatically adding the host key.
Command Execution: You can issue commands to networked devices using Paramiko and then retrieve the results. For running diagnostics, automating repetitive tasks, and retrieving device information, this feature is especially helpful.
The exec_command() method of the SSHClient class can be used to run a command through Paramiko. Stdin, Stdout, and Stderr are the three file−like objects that this method returns. The command's output can be viewed in stdout, and any errors can be addressed in stderr.
File Transfer: Using SFTP (Secure File Transfer Protocol), Paramiko enables file transfers between local and networked devices. Simple tasks like downloading log files or uploading configuration files are made easier by this feature.
For file transfer tasks, Paramiko offers the SFTPClient class. To transfer files to and from the remote device, you can use methods like put() and get().
Introduction to Netmiko
Network automation is made even simpler by Netmiko, a multi−vendor library built on top of Paramiko. Regardless of the vendor or operating system, it offers a unified API to communicate with different network devices. Among the many devices that Netmiko supports are those from Cisco, Juniper, Arista, Huawei, and other manufacturers.
To install Netmiko, you can use pip:
pip install netmiko
Simplifying Network Tasks with Netmiko
As a result of Netmiko's abstraction of network device differences, you can create network automation scripts that function consistently across different vendors. Here are some of Netmiko's salient characteristics:
Device Connection: A straightforward and reliable method of connecting to network devices is offered by Netmiko. The IP address, username, password, and optional SSH port of the device must all be provided in order to establish an SSH connection.
When establishing a connection, you have the option to specify the device type that Netmiko supports. For a Cisco IOS device, for instance, you could specify "cisco_ios" as the device type.
Command Execution: The send_command() method allows you to send commands to network devices. Retrieving command output is simple thanks to the way Netmiko handles SSH connections and command execution.
The command is passed as a parameter and the output is returned as a string by the send_command() method. Additional Netmiko methods, such as send_command_timing() and send_command_expect(), handle interactive commands and hold the output until certain prompts have been received.
Configuration Management: The send_config_from_file() method from Netmiko makes configuration management simpler. Device configurations can be kept in separate files, and Netmiko can be used to consistently apply these configurations to the devices.
With the help of this feature, you can automate configuration tasks like adding VLANs, setting up interfaces, and changing access control lists. You can easily manage and version control your network configurations by storing configurations in files.
Parsing Output: Send_command_timing() and send_command_expect() are built into Netmiko to parse command output. These techniques manage interactive commands and hold the output until a certain set of prompts has been issued.
Parsing command output is essential for obtaining pertinent data and carrying out additional operations based on the output. By offering methods that can handle various prompts and scenarios, Netmiko makes it simpler to automate difficult tasks.
Example: Retrieving Device Information
Let's take a practical example of using Paramiko and Netmiko to retrieve device information from a network device:
import paramiko from netmiko import ConnectHandler # Paramiko SSH connection ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect('192.168.0.1', username='admin', password='password') # Netmiko connection device = { 'device_type': 'cisco_ios', 'ip': '192.168.0.1', 'username': 'admin', 'password': 'password', } net_connect = ConnectHandler(**device) # Retrieve device information using Paramiko stdin, stdout, stderr = ssh.exec_command('show version') output = stdout.read().decode() print(output) # Retrieve device information using Netmiko output = net_connect.send_command('show version') print(output) # Close connections ssh.close() net_connect.disconnect()
In this example, we establish an SSH connection using Paramiko and Netmiko to retrieve the device's version information. Both methods provide similar functionality, but Netmiko simplifies the process by abstracting the SSH connection details and providing a unified API.
Conclusion
Paramiko and Netmiko are powerful Python libraries that simplify network tasks and enable efficient network automation. Whether you need to establish SSH connections, execute commands, transfer files, or manage configurations, these libraries provide high−level abstractions and consistent APIs. By leveraging their capabilities, you can streamline your network automation workflows and reduce manual effort. Incorporating Paramiko and Netmiko into your network automation arsenal empowers you to simplify and automate complex network tasks, making Python a valuable tool for network administrators and engineers. So, start exploring Paramiko and Netmiko to simplify your network tasks in Python and unleash the full potential of network automation.