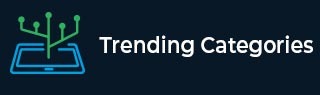
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Shortest Completing Word in Python
Suppose we have a dictionary words, and we have to find the minimum length word from a given dictionary words, it has all the letters from the string licensePlate. Now such a word is said to complete the given string licensePlate. Here, we will ignore case for letters. And it is guaranteed an answer exists. If there are more than one answers, then return answer that occurs first in the array.
The license plate there may be same letter occurring multiple times. So when a licensePlate of "PP", the word "pile" does not complete the licensePlate, but the word "topper" does.
So, if the input is like licensePlate = "1s3 PSt", words = ["step", "steps", "stripe", "stepple"], then the output will be "steps", as the smallest length word that contains the letters are "S", "P", "S", "T".
To solve this, we will follow these steps −
- alphabet := "abcdefghijklmnopqrstuvwxyz"
- letters := a list of s in lowercase by taking all s from licensePlate when s is in alphabet
- valid_words := a new list
- for each i in words, do
- append := True
- for each j in letters, do
- append := append and (number of j in letters <= number of j in i)
- if append is true, then
- insert i at the end of valid_words
- return minimum length word in valid_words
Let us see the following implementation to get better understanding −
Example
class Solution: def shortestCompletingWord(self, licensePlate, words): alphabet = "abcdefghijklmnopqrstuvwxyz" letters = [s.lower() for s in licensePlate if s.lower() in alphabet] valid_words = [] for i in words: append = True for j in letters: append = append and (letters.count(j) <= i.count(j)) if append: valid_words.append(i) return min(valid_words, key=len) ob = Solution() print(ob.shortestCompletingWord("1s3 PSt", ["step", "steps", "stripe", "stepple"]))
Input
"1s3 PSt", ["step", "steps", "stripe", "stepple"]
Output
steps