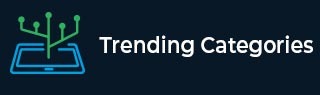
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
set vs unordered_set in C++ STL(3)
In this article, let us understand what is set and unordered_set in C++ STL and thereby gain knowledge of difference between them.
What is set?
A set is an Associative container which contains a sorted set of unique objects of type Key. Each element may occur only once, so duplicates are not allowed. A user can create a set by inserting elements in any order and the set will return a sorted data to the user which means set contains definition for sorting the data which is abstracted from the user.
The main reasons when set can be used are −
When sorted data is required
When duplicate values are not required only unique data is needed
When we want to use Binary Search Tree instead of Hash Table.
When there is no issue with search time as it takes log(n) complexity in searching
Input −
set = {2, 1, 5, 6, 9, 3, 2}
Output −
1, 2, 3, 5, 6, 9
Note − values are inserted in random order, but they are sorted by the set and also duplicate values are removed from the set.
Example
#include <iostream> #include <set> using namespace std; int main(){ //creating an array int arr[] = {2, 1, 5, 6, 9, 3, 2}; int size = sizeof(arr)/ sizeof(arr[0]); //declaring a set set<int> SET; //inserting elements from an array to set using insert() for(int i = 0; i<size; i++){ SET.insert(arr[i]); } set<int>::iterator it; cout<<"Values in set are: "; for(it = SET.begin(); it != SET.end(); it++){ cout <<*it<<" "; } }
Output
Output for the above code will be −
Values in set are: 1 2 3 5 6 9
What is unordered_set?
An unordered_set is an Associative container that contains an unordered set of data inserted randomly. Each element may occur only once, so duplicates are not allowed. A user can create an unordered set by inserting elements in any order and an unordered set will return data in any order i.e. unordered form.
The main reasons when unordered set can be used are −
When no sorted data is required means the data is available in an unordered format
When duplicate values are not required only unique data is needed
When we want to use Hash Table instead of a Binary Search Tree.
When a faster search is required as it take O(1) in Average case and O(n) in worst case
Input −
set = {2, 1, 5, 6, 9, 3, 2}
Output −
3, 9, 6, 5, 2
Example
#include <iostream> #include <unordered_set> using namespace std; int main (){ int arr[] = { 2, 1, 5, 6, 9, 3, 2 }; int size = sizeof (arr) / sizeof (arr[0]); unordered_set < int >U_SET; //inserting elements from an array to an unordered_set using insert() for (int i = 0; i < size; i++){ U_SET.insert (arr[i]); } unordered_set < int >::iterator it; cout << "Values in unordred set are: "; for (it = U_SET.begin (); it != U_SET.end (); it++){ cout << *it << " "; } }
Output
Output for the above code will be −
Values in unordered set are: 3 6 5 9 2 1