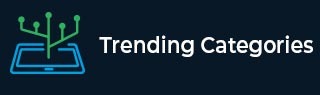
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Sending Email Using Smtp in Golang
In this golang article, we can send email using SMTP’s SendMail method,as well as using SMTP with go mail method,
SMTP stands for simple mail transfer protocol. This protocol is used to send messages between the servers. The net/smtp package provides a medium to send messages hence it must be imported in the program.
Syntax
smtp.PlainAuth()
This function belongs to the smtp and is primarily used to authenticate with the SMTP server using plain authentication.
smtp.SendMail()
This function is present in the SMTP package. It is used to send the message from the SMTP server.
smtpClient.Auth()
It is a method in the gomail package. It is used to authenticate with the SMTP server using different authentication methods.
smtpClient.StartTLS()
This method belongs to the gomail package and is used to establish a TLS session with the SMTP server
gomail.Send()
This method belongs to gomail package. It is used to send the email to the destination with the help of SMTP client.
Method 1
In this method, we will create a smtp server, smtp port, sender’s email, sender’s password and recipient email. Then, a message will be prepared which is to be sent using SMTP.
Algorithm
Step 1 − Create a package main and declare fmt(format package) net/smtp package in the program where main produces executable codes and fmt helps in formatting input and output.
Step 2 − Create a main function and in that function create a smtp server and smtp port
Step 3 − In this step, set the sender’s email, sender’s password and also the recipient’s email
Step 4 − Then, use byte function to set the message body by adding the recipient’s email, subject in it
Step 5 − Use PlainAuth method to authenticate with the SMTP server with sender email and password as inputs
Step 6 − Finally, send the email using SendMail function and if any error is encountered while sending the mail print it using panic
Step 7 − If no error is obtained print email is sent successfully
Example
Following is the Golang program to send email using SMTP with the help of SendMail method from smtp package
package main import ( "net/smtp" "fmt" ) func main() { smtp_server := "smtp.gmail.com" smtp_port := "587" senders_email := "kanishk_134@gmail.com" senders_password := "your_gmail_password" recipient_email := "recipient_email@Example.com" message := []byte("To: " + recipient_email + "\r\n" + "Subject: Go SMTP Test\r\n" + "\r\n" + "Hello,\r\n" + "This is a test email sent from Go!\r\n") auth := smtp.PlainAuth("", senders_email, senders_password, smtp_server) err := smtp.SendMail(smtp_server+":"+smtp_port, auth, senders_email, []string{recipient_email}, message) if err != nil { panic(err) } fmt.Println("Email sent successfully!") }
Output
Email sent successfully!
Method 2
In this particular method, a gomail object will be created to send the message using SMTP. It provides advanced features like HTML content, email templates and attachments.
Algorithm
Step 1 − Create a package main and declare fmt(format package) net/smtp and "gopkg.in/gomail.v2" package in the program where main produces executable codes and fmt helps in formatting input and output.
Step 2 − In the main function set the smtp server and smtp port to send the email
Step 3 − Then, set the sender’s email, sender’s password and recipient’s email
Step 4 − In this step, create an object using gomail.NewMessage and use Headers to create message to be sent to the receiver
Step 5 − Authenticate the SMTP server with sender’s email and sender’s password
Step 6 − Set the SMTP client using the Dial function from the SMTP package
Step 7 − Use Auth method to set up the authentication credentials
Step 8 − Call smtpClient.StartTLS(nil) to enable TLS encryption and then finally send the message using the gomail.Send function
Step 9 − If any error is encountered while sending message use panic to print the error
Step 10 − If no error is seen, print the email is sent successfully
Example
Following is the Golang program to send email using SMTP with the help of a gomail object
package main import ( "fmt" "net/smtp" "gopkg.in/gomail.v2" ) func main() { smtp_server := "smtp.gmail.com" smtp_port := 587 sender_email := "Rahul_email@gmail.com" sender_password := "ABC_email_password" recipient_email := "recipient_email@Example.com" message := gomail.NewMessage() message.SetHeader("From", sender_email) message.SetHeader("To", recipient_email) message.SetHeader("Subject", "Go SMTP Test") message.SetBody("text/plain", "Hello,\r\nThis is a test email sent from Go using the gomail package!") auth := smtp.PlainAuth("", sender_email, sender_password, smtp_server) smtpClient, err := smtp.Dial(smtp_server + ":" + string(smtp_port)) if err != nil { panic(err) } defer smtpClient.Close() smtpClient.Auth(auth) smtpClient.StartTLS(nil) err = gomail.Send(smtpClient, message) if err != nil { panic(err) } fmt.Println("Email sent successfully!") }
Output
Email sent successfully!
Conclusion
We compiled and executed the program of sending email with SMTP. In the first Example, we prepared the message using byte function and sent it using Send method and in the second Example we created a gomail object to send the message.