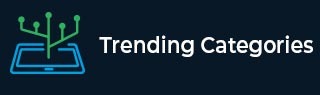
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Selenium WebDriver and DropDown Boxes.
We can handle dropdown with Selenium webdriver. The static dropdown in Selenium is handled with the Select class and the dropdown should be identified in the html code with the <select> tag.
Let us see the html code of a static dropdown.
We have to add the import org.openqa.selenium.support.ui.Select statement in the code to work with the methods available in the Select class. The methods to select an option from the dropdown are listed below −
selectByValue(val) – The option is selected whose value attribute matches with the argument passed to the method. This method can be used only if the dropdown option has the value attribute in its html code.
Syntax: Select sl = new Select (driver.findElement(By.name("name"))); sl.selectByValue ('value');
selectByVisibleText(txt) – The option is selected whose visible text matches with the argument passed to the method.
Syntax: Select sl = new Select (driver.findElement(By.name("name"))); sl.selectByVisibleText ('Selenium');
selectByIndex(n) – The option is selected whose index matches with the argument passed to the method. The index begins with zero.
Syntax: Select sl = new Select (driver.findElement(By.name("name"))); sl.selectByIndex(3);
Example
Code Implementation.
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; import org.openqa.selenium.support.ui.Select public class SelectDropDown{ public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "C:\Users\ghs6kor\Desktop\Java\chromedriver.exe"); WebDriver driver = new ChromeDriver(); String u = "https://www.tutorialspoint.com/selenium/selenium_automation_practice.htm" driver.get(u); driver.manage().timeouts().implicitlyWait(8, TimeUnit.SECONDS); // identify dropdown WebElement d=driver.findElement(By.xpath("//*[@name='continents']")); //dropdown handle with Select class Select sl = new Select(d); // select option with text visible sl.selectByVisibleText("Africa"); // select option with index sl.selectByIndex(4); driver.quit(); } }