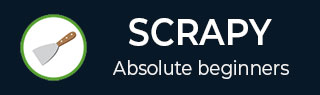
- Scrapy Tutorial
- Scrapy - Home
- Scrapy Basic Concepts
- Scrapy - Overview
- Scrapy - Environment
- Scrapy - Command Line Tools
- Scrapy - Spiders
- Scrapy - Selectors
- Scrapy - Items
- Scrapy - Item Loaders
- Scrapy - Shell
- Scrapy - Item Pipeline
- Scrapy - Feed exports
- Scrapy - Requests & Responses
- Scrapy - Link Extractors
- Scrapy - Settings
- Scrapy - Exceptions
- Scrapy Live Project
- Scrapy - Create a Project
- Scrapy - Define an Item
- Scrapy - First Spider
- Scrapy - Crawling
- Scrapy - Extracting Items
- Scrapy - Using an Item
- Scrapy - Following Links
- Scrapy - Scraped Data
- Scrapy Built In Services
- Scrapy - Logging
- Scrapy - Stats Collection
- Scrapy - Sending an E-mail
- Scrapy - Telnet Console
- Scrapy - Web Services
- Scrapy Useful Resources
- Scrapy - Quick Guide
- Scrapy - Useful Resources
- Scrapy - Discussion
Scrapy - Working with Items
Creating Items
You can create the items as shown in the following format −
>>myproduct = Product(name = 'Mouse', price = 400) >>print myproduct
The above code produces the following result −
Product(name = 'Mouse', price = 400)
Getting Field Values
You can get the field values as shown in the following way −
>>myproduct[name]
It will print result as 'Mouse'
Or in another way, you can get the value using get() method as −
>>myproduct.get(name)
It will print result as 'Mouse'
You can also check whether the field is present or not using the following way −
>>'name' in myproduct
It will print the result as 'True'
Or
>>'fname' in myproduct
It will print the result as 'False'
Setting Field Values
You can set value for the field shown as follows −
>>myproduct['fname'] = 'smith' >>myproduct['fname']
Accessing all Populated Values
It is possible to access all the values, which reside in the 'Product' item.
>>myproduct.keys()
It will print the result as −
['name', 'price']
Or you can access all the values along with the field values shown as follows −
>>myproduct.items()
It will print the result as −
[('name', 'Mouse'), ('price', 400)]
It's possible to copy items from one field object to another field object as described −
>> myresult = Product(myproduct) >> print myresult
It will print the output as −
Product(name = 'Mouse', price = 400)
>> myresult1 = myresult.copy() >> print myresult1
It will print the output as −
Product(name = 'Mouse', price = 400)