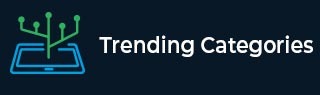
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Russian Doll Envelopes in C++
Suppose we have some envelops, these envelops has height and width values as pairs. We can put one envelop into another one if the height and width of second envelop both are smaller than the height and width of the first one. So, what will be the maximum number of envelops that we can put inside other. So, if the inputs are like [[5,5], [6,4], [6,8], [2,3]], then the output will be 3, as smallest envelop is [2,3], then [5,5], then [6,8].
To solve this, we will follow these steps −
- sort the array v based on height, when heights are same, compare with width
- if size of v is same as 0, then −
- return 0
- Define an array ret
- for initialize i := 0, when i < size of v, update (increase i by 1), do −
- Define an array temp = v[i]
- x := temp[1]
- low := 0, high := size of ret, curr := 0
- while low <= high, do −
- mid := low + (high - low) / 2
- if ret[mid] < temp[1], then −
- curr := mid + 1
- low := mid + 1
- Otherwise
- high := mid - 1
- if curr < 0, then −
- Ignore following part, skip to the next iteration
- if curr >= size of ret, then −
- insert temp[1] at the end of ret
- Otherwise
- ret[curr] := temp[1]
- return size of ret
Let us see the following implementation to get better understanding −
Example
#include <bits/stdc++.h> using namespace std; class Solution { public: static bool cmp(vector <int> a, vector <int> b){ if(a[0] == b[0])return a[1] > b[1]; return a[0] < b[0]; } int maxEnvelopes(vector<vector<int>>& v) { sort(v.begin(), v.end(), cmp); if(v.size() == 0)return 0; vector <int> ret; for(int i = 0; i < v.size(); i++){ vector <int> temp = v[i]; int x = temp[1]; int low = 0; int high = ret.size() -1; int curr = 0; while(low <= high){ int mid = low + (high - low) / 2; if(ret[mid]<temp[1]){ curr = mid + 1; low = mid + 1; }else{ high = mid - 1; } } if(curr < 0) continue; if(curr >= (int)ret.size()){ ret.push_back(temp[1]);; }else{ ret[curr] = temp[1]; } } return ret.size(); } }; main(){ Solution ob; vector<vector<int>> v = {{5,5}, {6,4}, {6,8}, {2,3}}; cout << (ob.maxEnvelopes(v)); }
Input
{{5,5}, {6,4}, {6,8}, {2,3}}
Output
3
Advertisements