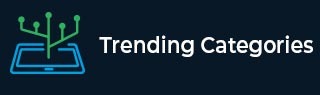
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Runtime Type Identification in Java
Runtime Type Identification in short RTTI is a feature that enables retrieval of the type of an object during run time. It is very crucial for polymorphism as in this oops functionality we have to determine which method will get executed. We can also implement it for primitive datatypes like Integers, Doubles and other datatypes. In this article, we will explain the use case of Runtime Type Identification in Java with the help of examples. Program for Runtime Type Identification Let’s discuss a few methods that can help us to identify type of object: instanceOf It is a comparison operator that checks whether an object is an instance of a specified class or not. Its return type is Boolean, if the object is an instance of a given class then it will return true otherwise false.
Syntax
nameOfObject instanceOf nameOfClass;
getClass()
It is a method of ‘java.lang’ package and is used to return the run time class of a specified object. It does not accept any arguments.
Syntax
nameOfObject.getClass();
It is the general syntax to call this method.
getName()
It returns the name of entities such as class, interface and primitives of specified objects of a given class. Its return type is String.
Syntax
nameOfClassobject.getName();
Example 1
The following example illustrates the use of instanceOf.
public class Main { public static void main(String[] args) { String st1 = "Tutorials Point"; if(st1 instanceof String) { System.out.println("Yes! st1 belongs to String"); } else { System.out.println("No! st1 not belongs to String"); } } }
Output
Yes! st1 belongs to String
In the above code, we have declared and initialized a String. The if-else block checked whether ‘st1’ is a String type or not using ‘instanceOf’.
Example 2
In the following example, we compare if two objects are of the same type or not using getClass() and getName() methods.
import java.util.*; class Student { String name; int regd; Student(String name, int regd) { // Constructor this.name = name; this.regd = regd; } } public class Main { public static void main(String[] args) { // creating objects of Class student Student st1 = new Student("Tutorialspoint", 235); Student st2 = new Student("Tutorix", 2011); // retrieving class name Class cls1 = st1.getClass(); Class cls2 = st2.getClass(); // checking whether name of objects are same or not if(cls1.getName() == cls2.getName()) { System.out.println("Both objects belongs to same class"); } else { System.out.println("Both objects not belongs to same class"); } } }
Output
Both objects belongs to same class
Example 3
In this particular example, we will declare and initialize two primitives and their corresponding wrapper classes. Then, using getClass() we will fetch the name of their classes.
public class Main { public static void main(String[] args) { double d1 = 50.66; int i1 = 108; // Wrapper class of double and integer Double data1 = Double.valueOf(d1); Integer data2 = Integer.valueOf(i1); System.out.println("Value of data1 = " + data1 + ", its type: " + data1.getClass()); System.out.println("Value of data2 = " + data2 + ", its type: " + data2.getClass()); } }
Output
Value of data1 = 50.66, its type: class java.lang.Double Value of data2 = 108, its type: class java.lang.Integer
Conclusion
In this article, we have explored Runtime Type Identification, it is more of a type introspection. We use this feature when we need to compare two objects and also during polymorphism as it allows us to retrieve pieces of information about classes, interfaces and so forth.