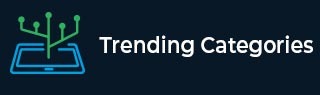
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Running multiple commands when a button is pressed in Tkinter
The Button widget provides a way to communicate through all the existing functionalities of an application. We can perform a certain action with the help of a Button that encapsulates the function and the objects. However, there might be cases when we want to perform multiple operations with a single button. This can be achieved by defining the lambda functions which target multiple events or callback in the application.
Example
In this example, we will add multiple events to a specific Button.
#Import the Tkinter Library from tkinter import * #Create an instance of Tkinter Frame win = Tk() #Set the geometry of window win.geometry("700x350") #Define functions def display_msg(): label.config(text="Top List of Programming Language") def show_list(): listbox= Listbox(win, height=10, width= 15, bg= 'grey', activestyle= 'dotbox',font='aerial') listbox.insert(1,"Go") listbox.insert(1,"Java") listbox.insert(1,"Python") listbox.insert(1,"C++") listbox.insert(1,"Ruby") listbox.pack() button.destroy() #Create a Label widget to display the message label= Label(win, text= "", font= ('aerial 18 bold')) label.pack(pady= 20) #Define a Button widget button= Button(win, text= "Click Here",command= lambda:[display_msg(), show_list()]) button.pack() win.mainloop()
Output
Running the above code will display a window containing a button.
When we click the Button, it will perform two tasks in parallel. It will display a window containing a Label widget and a List of strings.
Advertisements