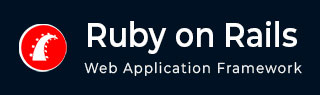
- Ruby on Rails Tutorial
- Ruby on Rails - Home
- Ruby on Rails - Introduction
- Ruby on Rails - Installation
- Ruby on Rails - Framework
- Ruby on Rails - Directory Structure
- Ruby on Rails - Examples
- Ruby on Rails - Database Setup
- Ruby on Rails - Active Records
- Ruby on Rails - Migrations
- Ruby on Rails - Controllers
- Ruby on Rails - Routes
- Ruby on Rails - Views
- Ruby on Rails - Layouts
- Ruby on Rails - Scaffolding
- Ruby on Rails - AJAX
- Ruby on Rails - File Uploading
- Ruby on Rails - Send Emails
- Ruby on Rails Resources
- Ruby on Rails - References Guide
- Ruby on Rails - Quick Guide
- Ruby on Rails - Resources
- Ruby on Rails - Discussion
- Ruby Tutorial
- Ruby Tutorial
Ruby on Rails - Nested with_scope
This examples shows how we can nest with_scope to fetch different results based on requirements.
# SELECT * FROM employees # WHERE (salary > 10000) # LIMIT 10 # Will be written as Employee.with_scope( :find => { :conditions => "salary > 10000", :limit => 10 }) do Employee.find(:all) end
Now, check another example how scope is cumulative.
# SELECT * FROM employees # WHERE ( salary > 10000 ) # AND ( name = 'Jamis' )) # LIMIT 10 # Will be written as Employee.with_scope( :find => { :conditions => "salary > 10000", :limit => 10 }) do Employee.find(:all) Employee.with_scope(:find => { :conditions => "name = 'Jamis'" }) do Employee.find(:all) end end
One more example which shows how previous scope is ignored.
# SELECT * FROM employees # WHERE (name = 'Jamis') # is written as Employee.with_scope( :find => { :conditions => "salary > 10000", :limit => 10 }) do Employee.find(:all) Employee.with_scope(:find => { :conditions => "name = 'Jamis'" }) do Employee.find(:all) end # all previous scope is ignored Employee.with_exclusive_scope(:find => { :conditions => "name = 'Jamis'" }) do Employee.find(:all) end end
rails-references-guide.htm
Advertisements