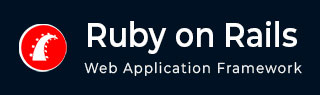
- Ruby on Rails Tutorial
- Ruby on Rails - Home
- Ruby on Rails - Introduction
- Ruby on Rails - Installation
- Ruby on Rails - Framework
- Ruby on Rails - Directory Structure
- Ruby on Rails - Examples
- Ruby on Rails - Database Setup
- Ruby on Rails - Active Records
- Ruby on Rails - Migrations
- Ruby on Rails - Controllers
- Ruby on Rails - Routes
- Ruby on Rails - Views
- Ruby on Rails - Layouts
- Ruby on Rails - Scaffolding
- Ruby on Rails - AJAX
- Ruby on Rails - File Uploading
- Ruby on Rails - Send Emails
- Ruby on Rails Resources
- Ruby on Rails - References Guide
- Ruby on Rails - Quick Guide
- Ruby on Rails - Resources
- Ruby on Rails - Discussion
- Ruby Tutorial
- Ruby Tutorial
Ruby on Rails - HTML Forms
Form
To create a form tag with the specified action, and with POST request, use the following syntax −
<%= form_tag :action => 'update', :id => @some_object %> <%= form_tag( { :action => :save, }, { :method => :post }) %>
Use :multipart => true to define a MIME-multipart form (for file uploads).
<%= form_tag( {:action => 'upload'}, :multipart => true ) %>
File Upload
Define a multipart form in your view −
<%= form_tag( { :action => 'upload' }, :multipart => true ) %> Upload file: <%= file_field( "form", "file" ) %> <br /> <%= submit_tag( "Upload file" ) %> <%= end_form_tag %>
Handle the upload in the controller −
def upload file_field = @params['form']['file'] rescue nil # file_field is a StringIO object file_field.content_type # 'text/csv' file_field.full_original_filename ... end
Text Fields
To create a text field use the following syntax −
<%= text_field :modelname, :attribute_name, options %>
Have a look at the following example −
<%= text_field "person", "name", "size" => 20 %>
This will generate following code −
<input type = "text" id = "person_name" name = "person[name]" size = "20" value = "<%= @person.name %>" />
To create hidden fields, use the following syntax;
<%= hidden_field ... %>
To create password fields, use the following syntax;
<%= password_field ... %>
To create file upload fields, use the following syntax;
<%= file_field ... %>
Text Area
To create a text area, use the following syntax −
<%= text_area ... %>
Have a look at the following example −
<%= text_area "post", "body", "cols" => 20, "rows" => 40%>
This will generate the following code −
<textarea cols = "20" rows = "40" id = "post_body" name =" post[body]"> <%={@post.body}%> </textarea>
Radio Button
To create a Radio Button, use the following syntax −
<%= radio_button :modelname, :attribute, :tag_value, options %>
Have a look at the following example −
radio_button("post", "category", "rails") radio_button("post", "category", "java")
This will generate the following code −
<input type = "radio" id = "post_category" name = "post[category]" value = "rails" checked = "checked" /> <input type = "radio" id = "post_category" name = "post[category]" value = "java" />
Checkbox Button
To create a Checkbox Button use the following syntax −
<%= check_box :modelname, :attribute,options,on_value,off_value%>
Have a look at the following example −
check_box("post", "validated")
This will generate the following code −
<input type = "checkbox" id = "post_validate" name = "post[validated]" value = "1" checked = "checked" /> <input name = "post[validated]" type = "hidden" value = "0" />
Let's check another example −
check_box("puppy", "gooddog", {}, "yes", "no")
This will generate following code −
<input type = "checkbox" id = "puppy_gooddog" name = "puppy[gooddog]" value = "yes" /> <input name = "puppy[gooddog]" type = "hidden" value = "no" />
Options
To create a dropdopwn list, use the following syntax −
<%= select :variable,:attribute,choices,options,html_options%>
Have a look at the following example −
select("post", "person_id", Person.find(:all).collect {|p| [ p.name, p.id ] })
This could generate the following code. It depends on what value is available in your database. −
<select name = "post[person_id]"> <option value = "1">David</option> <option value = "2">Sam</option> <option value = "3">Tobias</option> </select>
Date Time
Following is the syntax to use data and time −
<%= date_select :variable, :attribute, options %> <%= datetime_select :variable, :attribute, options %>
Following are examples of usage −
<%=date_select "post", "written_on"%> <%=date_select "user", "birthday", :start_year => 1910%> <%=date_select "user", "cc_date", :start_year => 2005, :use_month_numbers => true, :discard_day => true, :order => [:year, :month]%> <%=datetime_select "post", "written_on"%>
End Form Tag
Use following syntax to create </form> tag −
<%= end_form_tag %>