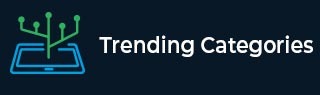
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Returning Value from a Subroutine in Perl
You can return a value from Perl subroutine as you do in any other programming language. If you are not returning a value from a subroutine then whatever calculation is last performed in a subroutine is automatically also the return value.
You can return arrays and hashes from the subroutine like any scalar but returning more than one array or hash normally causes them to lose their separate identities. So we will use references ( explained in the next chapter ) to return an array or hash from a function.
Example
Let's try the following example, which takes a list of numbers and then returns their average −
#!/usr/bin/perl # Function definition sub Average { # get total number of arguments passed. $n = scalar(@_); $sum = 0; foreach $item (@_) { $sum += $item; } $average = $sum / $n; return $average; } # Function call $num = Average(10, 20, 30); print "Average for the given numbers : $num\n";
Output
When the above program is executed, it produces the following result −
Average for the given numbers : 20
Advertisements