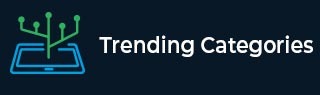
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Returning an array with the minimum and maximum elements JavaScript
Provided an array of numbers, let’s say −
const arr = [12, 54, 6, 23, 87, 4, 545, 7, 65, 18, 87, 8, 76];
We are required to write a function that picks the minimum and maximum element from the array and returns an array of those two numbers with minimum at index 0 and maximum at 1.
We will use the Array.prototype.reduce() method to build a minimum maximum array like this −
Example
const arr = [12, 54, 6, 23, 87, 4, 545, 7, 65, 18, 87, 8, 76]; const minMax = (arr) => { return arr.reduce((acc, val) => { if(val < acc[0]){ acc[0] = val; } if(val > acc[1]){ acc[1] = val; } return acc; }, [Infinity, -Infinity]); }; console.log(minMax(arr));
Output
The output in the console will be −
[ 4, 545 ]
Advertisements