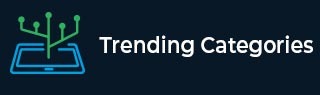
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Return the difference between the maximum & minimum number formed out of the number n in JavaScript
We have to write a function maximumDifference() that takes in a positive number n and returns the difference between the maximum number and the minimum number that can be formed out of the number n.
For example −
If the number n is 203,
The maximum number that can be formed from its digits will be 320
The minimum number that can be formed from its digits will be 23 (placing the zero at one’s place)
And the difference will be −
320-23 = 297
Therefore, the output should be 297
Let's write the code for this function −
Example
const digitDifference = num => { const asc = +String(num).split("").sort((a, b) => { return (+a) - (+b); }).join(""); const des = +String(num).split("").sort((a, b) => { return (+b) - (+a); }).join(""); return des - asc; }; console.log(digitDifference(203)); console.log(digitDifference(123)); console.log(digitDifference(546)); console.log(digitDifference(2354));
Output
The output in the console will be −
297 198 198 3087
Advertisements