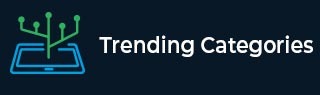
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Return a new array of given shape and type without initializing entries in Numpy
To return a new array of given shape and type, without initializing entries, use the ma.empty() method in Python Numpy. The 1st parameter sets the shape of the empty array. The dtype parameter sets the desired output data-type for the array. The method returns an array of uninitialized (arbitrary) data of the given shape, dtype, and order. Object arrays will be initialized to None.
Steps
At first, import the required library −
import numpy as np import numpy.ma as ma
Return a new array of given shape and type, without initializing entries using the ma.empty() method in Python Numpy −
arr = ma.empty((5, 5),dtype = np.int32)
Displaying our array −
print("Array...
",arr)
Get the dimensions of the Array −
print("
Array Dimensions...
",arr.ndim)
Get the shape of the Array −
print("
Our Array Shape...
",arr.shape)
Get the number of elements of the Array −
print("
Elements in the Array...
",arr.size)
Example
# Python ma.MaskedArray - Return a new array of given shape and type without initializing entries import numpy as np import numpy.ma as ma # To return a new array of given shape and type, without initializing entries, use the ma.empty() method in Python Numpy # The 1st parameter sets the shape of the empty array # The dtype parameter sets the desired output data-type for the array arr = ma.empty((5, 5),dtype = np.int32) # Displaying our array print("Array...
",arr) # Get the dimensions of the Array print("
Array Dimensions...
",arr.ndim) # Get the shape of the Array print("
Our Array Shape...
",arr.shape) # Get the number of elements of the Array print("
Elements in the Array...
",arr.size)
Output
Array... [[ 0 0 0 0 117] [ 99 104 32 102 105] [108 101 32 111 114] [ 32 100 105 114 101] [ 99 116 111 114 121]] Array Dimensions... 2 Our Array Shape... (5, 5) Elements in the Array... 25
Advertisements