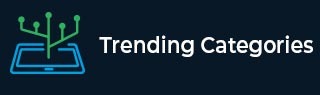
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Replace each node with its Surpasser Count in Linked List Using C++
We are given a linked list, and we need to find elements in the given linked list which are greater and present in the right of the current element. The count of these elements needs to be substituted into the current node's value.
Let's take a linked list with the following characters and replace each node with its Surpasser count −
4 -> 6 -> 1 -> 4 -> 6 -> 8 -> 5 -> 8 -> 3
From backward, traverse the linked list (so we do not need to worry about elements to the left of the current). Our data structure keeps track of current elements in sorted order. Replace the current element in our sorted data structure with the total number of elements above it.
By the recursion method, the linked list will be traversed backward. An alternative is PBDS. The use of PBDS allows us to find elements that are strictly smaller than a certain key. We can add the current element and subtract it from an element that is strictly smaller.
PBDS does not allow duplicate elements. However, we need duplicate elements for counting. In order to make each entry unique, we will insert a pair in PBDS (first = element, second = index). To find total elements equal to the current element, we will then use a hash map. A hashmap stores the number of occurrences of each element (a basic integer to integer map).
Example
Following is the C++ program to replace each node in a linked list with its surpasser count −
#include <iostream> #include <unordered_map> #include <ext/pb_ds/assoc_container.hpp> #include <ext/pb_ds/tree_policy.hpp> #define oset tree<pair<int, int>, null_type,less<pair<int, int>>, rb_tree_tag, tree_order_statistics_node_update> using namespace std; using namespace __gnu_pbds; class Node { public: int value; Node * next; Node (int value) { this->value = value; next = NULL; } }; void solve (Node * head, oset & os, unordered_map < int, int >&mp, int &count){ if (head == NULL) return; solve (head->next, os, mp, count); count++; os.insert ( { head->value, count} ); mp[head->value]++; int numberOfElements = count - mp[head->value] - os.order_of_key ({ head->value, -1 }); head->value = numberOfElements; } void printList (Node * head) { while (head) { cout << head->value << (head->next ? "->" : ""); head = head->next; } cout << "\n"; } int main () { Node * head = new Node (43); head->next = new Node (65); head->next->next = new Node (12); head->next->next->next = new Node (46); head->next->next->next->next = new Node (68); head->next->next->next->next->next = new Node (85); head->next->next->next->next->next->next = new Node (59); head->next->next->next->next->next->next->next = new Node (85); head->next->next->next->next->next->next->next->next = new Node (37); oset os; unordered_map < int, int >mp; int count = 0; printList (head); solve (head, os, mp, count); printList (head); return 0; }
Output
43->65->12->46->68->85->59->85->30 6->3->6->4->2->0->1->0->0
Explanation
So for 1st element, element = [65, 46, 68, 85, 59, 85] which is 6
2nd element, element = [68, 85, 85] which is 3
And so on for all elements
Conclusion
This problem requires some understanding of data structures and recursion. We need to list down the approach, and then from observation and knowledge, we deduce a data structure for our requirement. If you like this article, read more and stay tuned.